用C语言设计计算长方形的面积,使用源代码插桩法进行测试长方形的边长不能为负数 截图
时间: 2024-12-15 19:17:50 浏览: 6
在C语言中,我们可以首先编写一个函数用于计算长方形的面积,然后使用源代码插桩(Source Code Instrumentation)技术,这是一种动态代码修改的技术,在代码中插入检查边长是否为负数的条件。这里是一个简单的示例:
```c
#include <stdio.h>
// 定义长方形结构体
typedef struct {
float length;
float width;
} Rectangle;
// 计算长方形面积的原函数
float calculate_area(Rectangle rect) {
return rect.length * rect.width;
}
// 插桩后的函数,添加对边长非负的检查
float instrumented_calculate_area(Rectangle rect) {
if (rect.length <= 0 || rect.width <= 0) {
printf("Error: 长度或宽度不能为负数!\n");
return 0; // 或者你可以选择抛出错误或返回特殊值
}
return calculate_area(rect);
}
int main() {
Rectangle rectangle = {5.0, 3.0}; // 正常情况下的边长
float area = instrumented_calculate_area(rectangle); // 调用插桩后的函数
if (area > 0) {
printf("长方形的面积是: %.2f\n", area);
}
return 0;
}
```
在这个例子中,`instrumented_calculate_area()` 函数就是插桩后的版本,它在计算面积前会先检查长度和宽度是否大于0。如果发现边长为负,程序会打印错误信息并停止计算。
阅读全文
相关推荐
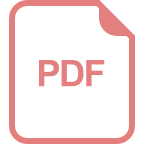
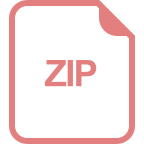


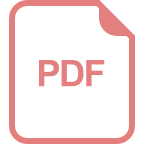








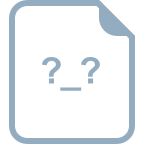
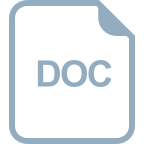


