详细讲解这段在C#中的代码 private CpuType cpuType = new CpuType();,特别是new的使用方法和原理
时间: 2024-04-18 07:32:46 浏览: 48
在C#中,关键字 `new` 用于创建一个对象的实例。在给定的代码中,`private CpuType cpuType = new CpuType();` 创建了一个名为 `cpuType` 的私有变量,并将其初始化为 `CpuType` 类的一个新实例。
`new CpuType()` 表示调用 `CpuType` 类的构造函数来创建一个对象实例。构造函数是一个特殊的方法,用于在创建对象时进行初始化操作。在这个例子中,`CpuType` 类可能有一个无参数的默认构造函数,该构造函数在创建对象时会执行一些默认的初始化操作。
通过使用 `new` 关键字,我们可以实例化一个类,并将其分配给变量 `cpuType`。这样,我们就可以通过 `cpuType` 变量来访问和操作 `CpuType` 类的成员(属性、方法等)。
需要注意的是,`private` 关键字表示 `cpuType` 变量是一个私有成员,只能在当前类内部访问。这意味着其他类无法直接访问或修改 `cpuType` 变量的值,除非提供了相应的公开接口或方法。
总结起来,给定的代码在C#中创建了一个名为 `cpuType` 的私有变量,并将其初始化为 `CpuType` 类的一个新实例。这样可以在当前类内部使用和操作 `cpuType` 对象。
相关问题
C# S7通信类库代码
以下是一个简单的C# S7通信类库代码示例:
```csharp
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using S7.Net;
namespace S7Communication
{
public class S7Comm
{
private Plc _plc;
public S7Comm(string ipAddress, int rack, int slot)
{
_plc = new Plc(CpuType.S71200, ipAddress, rack, slot);
}
public bool Connect()
{
try
{
_plc.Open();
return true;
}
catch (Exception ex)
{
Console.WriteLine(ex.Message);
return false;
}
}
public bool Disconnect()
{
try
{
_plc.Close();
return true;
}
catch (Exception ex)
{
Console.WriteLine(ex.Message);
return false;
}
}
public bool ReadBool(string address)
{
try
{
bool value = _plc.ReadBool(address);
return value;
}
catch (Exception ex)
{
Console.WriteLine(ex.Message);
return false;
}
}
public bool WriteBool(string address, bool value)
{
try
{
_plc.Write(address, value);
return true;
}
catch (Exception ex)
{
Console.WriteLine(ex.Message);
return false;
}
}
}
}
```
c# S7.net 通信代码
以下是使用S7.net库进行C#和S7-1200 PLC通信的示例代码:
```csharp
using System;
using System.Windows.Forms;
using S7.Net;
namespace S7Comm
{
public partial class MainForm : Form
{
private Plc _plc;
private Timer _timer;
public MainForm()
{
InitializeComponent();
// 初始化定时器
_timer = new Timer();
_timer.Interval = 1000;
_timer.Tick += Timer_Tick;
}
private void MainForm_Load(object sender, EventArgs e)
{
try
{
// 创建PLC对象
_plc = new Plc(CpuType.S71200, "192.168.0.1", 0, 1);
// 连接PLC
_plc.Open();
// 启动定时器
_timer.Start();
}
catch (Exception ex)
{
MessageBox.Show($"连接PLC失败:{ex.Message}");
}
}
private void MainForm_FormClosing(object sender, FormClosingEventArgs e)
{
// 关闭定时器
_timer.Stop();
// 断开PLC连接
if (_plc != null && _plc.IsConnected)
{
_plc.Close();
}
}
private void Timer_Tick(object sender, EventArgs e)
{
try
{
// 读取M0.0的值
bool value = _plc.ReadBool("M0.0");
// 更新UI
if (value)
{
labelStatus.Text = "开";
}
else
{
labelStatus.Text = "关";
}
}
catch (Exception ex)
{
MessageBox.Show($"读取PLC数据失败:{ex.Message}");
}
}
}
}
```
在上面的代码中,我们使用`Plc`类来连接S7-1200 PLC,并使用定时器来周期性地读取一个M位的值(M0.0)。
请注意,要使用S7.net库进行通信,需要在项目中添加对S7.Net.dll的引用。可以从S7.net库的GitHub页面上下载并安装它。
阅读全文
相关推荐
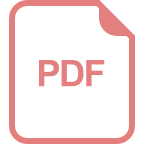
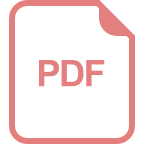
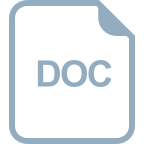
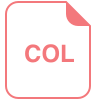
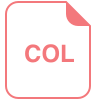
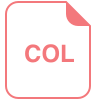
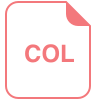
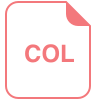
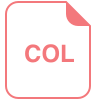
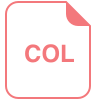
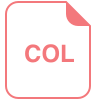
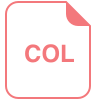
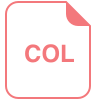
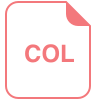
