如何python绘制函数调用关系图
时间: 2024-10-08 08:07:36 浏览: 20
在Python中,你可以使用`networkx`库来绘制函数调用关系图。`networkx`是一个非常强大的图形处理工具,特别适合于表示复杂的网络结构,包括函数间的依赖关系。以下是一个简单的步骤指南:
1. 首先,你需要安装`networkx`和`matplotlib`库,如果还没安装可以使用`pip`安装:
```
pip install networkx matplotlib
```
2. 导入需要的模块:
```python
import networkx as nx
import matplotlib.pyplot as plt
from inspect import getmembers, isfunction
```
3. 定义一个函数来获取所有的函数调用信息:
```python
def get_function_calls(obj):
members = getmembers(obj, predicate=isfunction)
calls = [(caller, callee) for caller, callee in members if callee.__name__ != '__init__']
return calls
```
4. 使用这个函数收集你想要分析的对象的所有函数调用:
```python
target_module = your_module # 替换为你感兴趣的模块名
function_calls = get_function_calls(target_module)
```
5. 创建一个图并添加节点和边:
```python
G = nx.DiGraph() # 使用有向图来表示函数调用
for caller, callee in function_calls:
G.add_edge(caller.__name__, callee.__name__)
pos = nx.spring_layout(G) # 自动布局节点位置
```
6. 绘制图形:
```python
plt.figure(figsize=(10, 8))
nx.draw_networkx_nodes(G, pos, node_size=800, node_color='lightblue')
nx.draw_networkx_edges(G, pos, arrows=True)
nx.draw_networkx_labels(G, pos, font_weight='bold', font_family='sans-serif')
plt.title('Function Call Graph')
plt.show()
```
这将展示出目标模块中函数之间的调用关系。记得替换`your_module`为实际的模块名称。
相关推荐
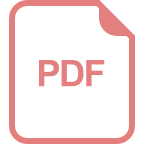
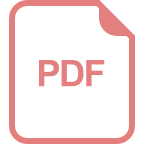
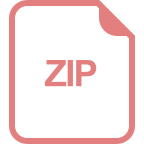





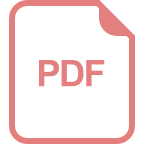
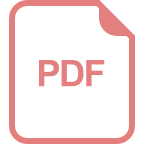
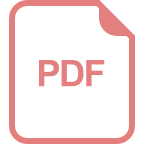
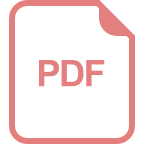
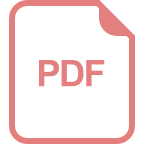




