python数据分组
时间: 2023-08-15 10:04:09 浏览: 68
在Python中,可以使用一些方法来对数据进行分组。以下是几种常见的方法:
1. 使用字典:
可以使用字典来将数据分组,其中键表示组的标识,值表示属于该组的数据。遍历数据时,将每个元素添加到相应的组中。
```python
data = [1, 2, 3, 4, 5, 6]
groups = {}
for item in data:
key = item % 2 # 以奇偶性为标识进行分组
if key not in groups:
groups[key] = []
groups[key].append(item)
print(groups)
```
输出:
```
{1: [1, 3, 5], 0: [2, 4, 6]}
```
2. 使用列表推导式和条件表达式:
可以使用列表推导式和条件表达式将数据分组。
```python
data = [1, 2, 3, 4, 5, 6]
groups = [[item for item in data if item % 2 == 0], [item for item in data if item % 2 != 0]]
print(groups)
```
输出:
```
[[2, 4, 6], [1, 3, 5]]
```
3. 使用itertools.groupby函数:
`itertools.groupby` 函数可以根据指定的条件对数据进行分组。需要注意的是,数据在进行分组前需要先排序。
```python
import itertools
data = [1, 2, 3, 4, 5, 6]
sorted_data = sorted(data)
groups = [list(group) for key, group in itertools.groupby(sorted_data, lambda x: x % 2)]
print(groups)
```
输出:
```
[[2, 4, 6], [1, 3, 5]]
```
以上是几种常见的方法,你可以根据具体的需求选择合适的方法进行数据分组。
相关推荐
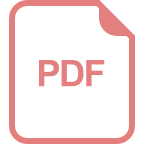
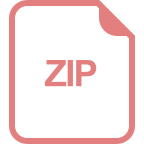
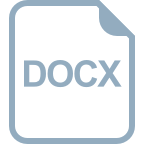














