使用谱聚类算法分割与k均值聚类算法分割图像进行对比,并显示轮廓
时间: 2023-07-17 18:11:26 浏览: 54
好的,我们可以使用轮廓来比较谱聚类算法和K均值聚类算法在图像分割上的效果。下面是使用Python进行对比并显示轮廓的示例代码:
```python
import numpy as np
from sklearn.cluster import KMeans, SpectralClustering
from skimage.segmentation import mark_boundaries
from skimage.io import imread, imshow
import matplotlib.pyplot as plt
from skimage.measure import find_contours
# 读取图像
image = imread('image.jpg')
# 将图像转换为特征向量
features = image.reshape(-1, 3)
# 使用K均值聚类算法进行图像分割
kmeans = KMeans(n_clusters=5, random_state=0)
kmeans_labels = kmeans.fit_predict(features)
# 使用谱聚类算法进行图像分割
clustering = SpectralClustering(n_clusters=5, affinity='nearest_neighbors', random_state=0)
spectral_labels = clustering.fit_predict(features)
# 计算K均值聚类算法的轮廓
kmeans_contours = find_contours(kmeans_labels.reshape(image.shape[:2]), 0.5)
# 计算谱聚类算法的轮廓
spectral_contours = find_contours(spectral_labels.reshape(image.shape[:2]), 0.5)
# 将分割结果可视化并显示轮廓
plt.subplot(131)
imshow(image)
plt.title('Original Image')
plt.subplot(132)
kmeans_segmented_image = mark_boundaries(image, kmeans_labels.reshape(image.shape[:2]))
imshow(kmeans_segmented_image)
plt.title('K-means Segmented Image')
for contour in kmeans_contours:
plt.plot(contour[:, 1], contour[:, 0], 'r-', linewidth=2)
plt.subplot(133)
spectral_segmented_image = mark_boundaries(image, spectral_labels.reshape(image.shape[:2]))
imshow(spectral_segmented_image)
plt.title('Spectral Segmented Image')
for contour in spectral_contours:
plt.plot(contour[:, 1], contour[:, 0], 'r-', linewidth=2)
plt.tight_layout()
plt.show()
```
在上述代码中,我们首先使用K均值聚类算法和谱聚类算法分别对图像进行分割,并得到对应的聚类标签。然后,使用`find_contours`函数计算出每个聚类的轮廓。最后,我们使用`mark_boundaries`函数将分割结果标记在原始图像上,并通过`plot`函数将轮廓绘制出来。
通过比较K均值聚类算法和谱聚类算法的分割结果和轮廓,可以对两种算法的性能进行对比评估。
相关推荐
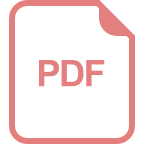
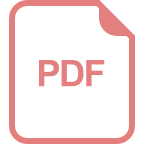
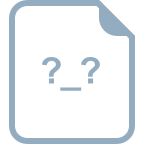














