python123题目个税计算器
时间: 2024-12-27 09:19:29 浏览: 2
### Python 实现个人所得税计算器
#### 题目描述
编写一个程序,输入用户的月收入金额,计算并输出应缴纳的个人所得税。
#### 解题思路
中国现行的个人所得税税率表采用累进制。根据不同的收入区间适用不同税率。具体税率如下:
| 应纳税所得额 | 税率(%) |
| --- | --- |
| 不超过3,000元的部分 | 3% |
| 超过3,000元至12,000元部分 | 10% |
| 超过12,000元至25,000元部分 | 20% |
| 超过25,000元至35,000元部分 | 25% |
| 超过35,000元至55,000元部分 | 30% |
| 超过55,000元至80,000元部分 | 35% |
| 超过80,000元部分 | 45% |
税前工资减去起征点(通常为5000元),得到应纳税所得额。再根据不同档次按比例计税[^1]。
#### 代码实现
下面是一个简单的Python函数来完成这个功能:
```python
def calculate_tax(income):
tax_start_point = 5000
taxable_income = income - tax_start_point
if taxable_income <= 0:
return 0
elif taxable_income <= 3000:
rate = 0.03
deduction = 0
elif taxable_income <= 12000:
rate = 0.10
deduction = 210
elif taxable_income <= 25000:
rate = 0.20
deduction = 1410
elif taxable_income <= 35000:
rate = 0.25
deduction = 2660
elif taxable_income <= 55000:
rate = 0.30
deduction = 4410
elif taxable_income <= 80000:
rate = 0.35
deduction = 7160
else:
rate = 0.45
deduction = 15160
tax = taxable_income * rate - deduction
actual_tax = max(tax, 0)
return round(actual_tax, 2)
income = float(input("请输入您的月薪:"))
tax_amount = calculate_tax(income)
print(f"您本月需缴交的个人所得税为 {tax_amount} 元")
```
阅读全文
相关推荐
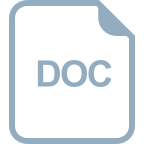
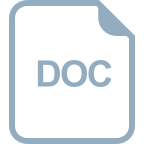
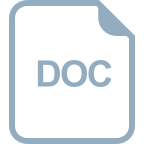

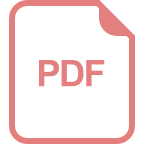

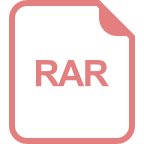
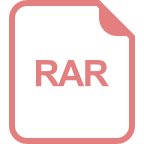


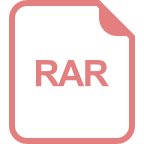
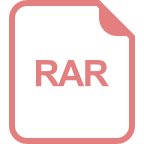
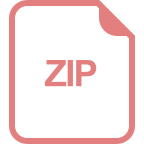
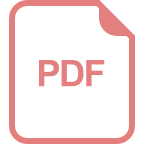