Java使用HttpClient5实现发送HTTP请求
时间: 2024-11-27 11:10:43 浏览: 14
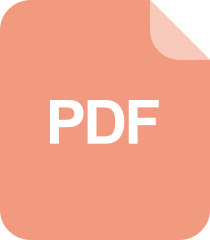
JAVA通过HttpClient发送HTTP请求的方法示例
在Java中,Apache HttpClient是一个常用的库,用于发送HTTP请求。版本5.x已经被弃用,推荐使用更现代的HTTP客户端如HttpClient 4.x或更先进的OkHttp。以下是使用HttpClient 4.x发送GET和POST请求的基本步骤:
1. **添加依赖**:
首先,你需要在你的项目中引入`org.apache.httpcomponents:httpclient`和`org.apache.httpcomponents:httpcore`库。
```java
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
<version>4.5.13</version> <!-- 最近的一个稳定版本 -->
</dependency>
```
2. **创建HttpClient实例**:
创建HttpClient对象并设置超时时间等配置。
```java
CloseableHttpClient httpClient = HttpClients.createDefault();
HttpGet httpGet = new HttpGet("http://example.com/api");
```
3. **发送GET请求**:
发起GET请求,并处理响应。
```java
HttpResponse response = httpClient.execute(httpGet);
try {
StatusLine statusLine = response.getStatusLine();
if (statusLine.getStatusCode() == HttpStatus.SC_OK) {
HttpEntity entity = response.getEntity();
// 这里可以读取响应内容
String responseBody = EntityUtils.toString(entity);
}
} finally {
response.close();
}
```
4. **发送POST请求**:
对于POST请求,需要构建一个`HttpPost`对象,并设置请求体。
```java
HttpPost httpPost = new HttpPost("http://example.com/api");
try {
List<NameValuePair> nameValuePairs = new ArrayList<>();
nameValuePairs.add(new BasicNameValuePair("key", "value"));
httpPost.setEntity(new UrlEncodedFormEntity(nameValuePairs));
HttpResponse response = httpClient.execute(httpPost);
// ...处理响应
} finally {
httpPost.releaseConnection();
}
```
5. **关闭连接**:
使用完毕后,记得关闭HttpClient连接。
```java
httpClient.close();
```
阅读全文
相关推荐
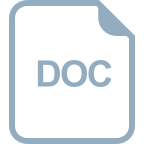
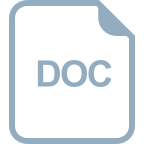
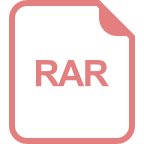
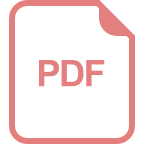
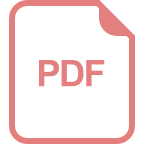
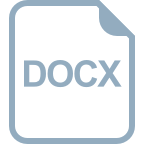
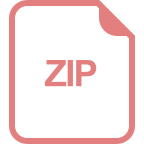
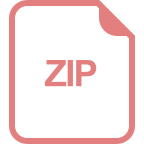
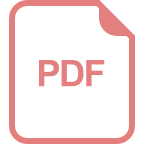
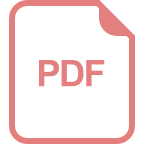
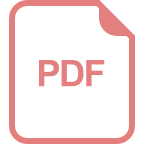
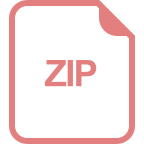
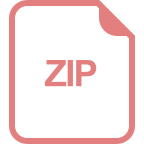
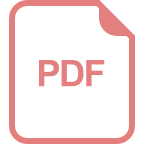

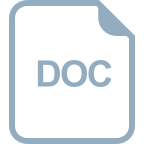
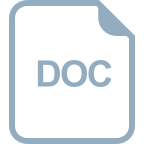