数据结构(C语言)--实现线性顺序表的查找、插入、删除、合并操作
时间: 2024-09-27 17:12:18 浏览: 33
在C语言中,线性顺序表是一种基础的数据结构,它通过数组的方式存储元素,每个元素的存储位置是连续的。以下是实现线性顺序表常用的基本操作:
1. **查找(Search)**:
- 定义:给定一个值和一个指向列表起始地址的指针,找到第一个等于该值的元素的位置。如果找不到则返回`-1`。
```c
int linear_search(int arr[], int size, int target) {
for (int i = 0; i < size; ++i) {
if (arr[i] == target) {
return i;
}
}
return -1;
}
```
2. **插入(Insertion)**:
- 插入一个新元素到指定位置(如果需要,可以调整后续元素的位置)。
```c
void insert(int arr[], int size, int index, int value) {
if (index >= 0 && index <= size) {
memmove(&arr[index + 1], &arr[index], sizeof(arr[0]) * (size - index));
arr[index] = value;
} else {
printf("Invalid index\n");
}
}
```
3. **删除(Deletion)**:
- 删除指定索引处的元素,并调整后续元素的索引。
```c
void delete(int arr[], int size, int index) {
if (index >= 0 && index < size - 1) {
memmove(&arr[index], &arr[index + 1], sizeof(arr[0]) * (size - 1));
} else {
printf("Invalid index\n");
}
}
```
4. **合并(Merge)**:
- 合并两个已排序的线性顺序表成一个新的已排序列表。这里假设你有两个独立的数组。
```c
void merge_sorted(int* list1, int* list2, int size1, int size2, int combined[]) {
int i = 0, j = 0, k = 0;
while (i < size1 && j < size2) {
if (list1[i] < list2[j]) {
combined[k++] = list1[i++];
} else {
combined[k++] = list2[j++];
}
}
// 将剩余未处理的元素添加到结果数组
while (i < size1) {
combined[k++] = list1[i++];
}
while (j < size2) {
combined[k++] = list2[j++];
}
}
```
以上操作都需要谨慎处理边界条件和内存移动。请注意,`memmove()`函数用于安全地移动数据,避免覆盖其他变量。
相关推荐
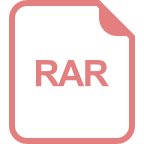
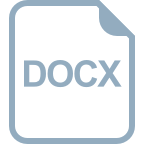
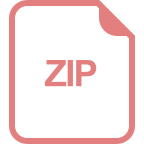















