用python设计一个算法,求一个循环单链表中值为y的结点的个数
时间: 2024-10-20 17:16:14 浏览: 18
在Python中,我们可以使用迭代的方式来设计一个算法来计算一个循环单链表中值为`y`的节点个数。首先,我们需要创建一个Node类表示链表节点,同时保持一个指针`current`指向当前节点以及一个标志`is_circular`来判断链表是否为循环。
```python
class Node:
def __init__(self, value):
self.value = value
self.next = None
def is_circular(head):
if head is None or head.next is None:
return False
slow = head
fast = head.next
while slow != fast:
if fast is None or fast.next is None:
return False
slow = slow.next
fast = fast.next.next
return True
def count_nodes_with_value(head, y):
current = head
count = 0
while True:
if current.value == y:
count += 1
if current is head and is_circular(current): # 如果找到环,继续从头开始计数
current = head
else:
current = current.next
if not current:
break # 链表结束
return count
# 使用示例:
# 定义链表节点
head = Node(1)
second_node = Node(2)
third_node = Node(3)
fourth_node = Node(4)
# 连接链表
head.next = second_node
second_node.next = third_node
third_node.next = fourth_node
fourth_node.next = head # 创建循环链表
# 求值为3的节点个数
node_count = count_nodes_with_value(head, 3)
print(f"值为{y}的节点个数是: {node_count}")
阅读全文
相关推荐
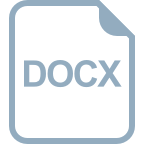
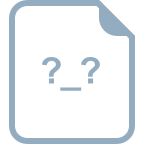
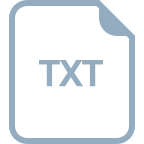












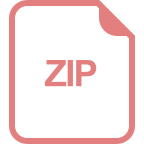
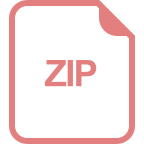