python aes文件加解密算法
时间: 2024-10-08 21:20:07 浏览: 38
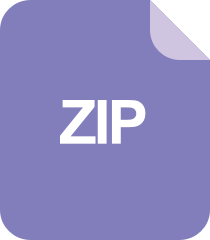
python之AES加密解密源码

在Python中,我们可以使用第三方库`cryptography`来进行AES(高级加密标准)文件的加解密操作。以下是基本步骤:
1. **安装依赖**:
首先需要通过pip安装`cryptography`库:
```
pip install cryptography
```
2. **导入模块**:
```python
from cryptography.hazmat.primitives.ciphers import Cipher, algorithms, modes
from cryptography.hazmat.backends import default_backend
from cryptography.hazmat.primitives.kdf.pbkdf2 import PBKDF2HMAC
from os import urandom
```
3. **生成密钥和初始向量(IV)**:
```python
password = b'some_password'
salt = urandom(16) # 可选,用于增强安全性的随机盐
kdf = PBKDF2HMAC(algorithm=algorithms.HMAC(algorithm=hashes.SHA256(), length=32),
salt=salt, length=32, iterations=100000, backend=default_backend())
key = kdf.derive(password)
iv = urandom(16) # AES-CTR模式下需要
```
4. **加密文件**:
```python
def encrypt_file(file_path, output_path, key, iv):
cipher = Cipher(algorithms.AES(key), modes.CTR(iv), backend=default_backend())
encryptor = cipher.encryptor()
with open(file_path, 'rb') as in_file:
with open(output_path, 'wb') as out_file:
for chunk in iter(lambda: in_file.read(24), b""):
encrypted_data = encryptor.update(chunk)
out_file.write(encrypted_data)
out_file.write(encryptor.finalize()) # 写入最终的填充块
```
5. **解密文件**:
```python
def decrypt_file(input_path, output_path, key, iv):
cipher = Cipher(algorithms.AES(key), modes.CTR(iv), backend=default_backend())
decryptor = cipher.decryptor()
with open(input_path, 'rb') as in_file:
with open(output_path, 'wb') as out_file:
while True:
encrypted_chunk = in_file.read(24)
if not encrypted_chunk:
break
decrypted_data = decryptor.update(encrypted_chunk)
out_file.write(decrypted_data)
out_file.write(decryptor.finalize()) # 写入解密后的数据
```
6. **示例用法**:
```python
encrypt_file('input.txt', 'output.enc', key, iv)
decrypt_file('output.enc', 'output_dec.txt', key, iv)
```
阅读全文