读取文件夹内的所有.dat文件,并画图。其中图的横坐标值为.dat文件的文件名
时间: 2024-09-25 12:19:53 浏览: 9
在Python中,我们可以使用os和pandas库来遍历文件夹,读取`.dat`文件,然后利用matplotlib来绘制图像。以下是大致的步骤:
1. 首先,确保已安装了必要的库:
```bash
pip install pandas matplotlib numpy os
```
2. 导入需要的库:
```python
import os
import pandas as pd
import matplotlib.pyplot as plt
import numpy as np
```
3. 定义函数来读取一个`.dat`文件:
```python
def read_dat_file(file_path):
return pd.read_csv(file_path, sep='\t', engine='python') if file_path.endswith('.dat') else None # 假设文件使用'\t'分隔
```
4. 遍历文件夹,读取每个`.dat`文件:
```python
data_frames = []
file_dir = 'your_directory' # 替换为你的文件夹路径
for filename in os.listdir(file_dir):
if filename.endswith('.dat'):
file_path = os.path.join(file_dir, filename)
df = read_dat_file(file_path)
if df is not None:
data_frames.append(df)
```
5. 将文件名转换为横坐标值,并准备绘图:
```python
file_names = [os.path.splitext(filename)[0] for filename in os.listdir(file_dir)]
x_values = list(range(len(data_frames))) # 使用文件数量作为简单横坐标
# 拟合一条线或者其他适合的图形。这里以直线为例:
y_values = [np.random.rand() * len(df) for df in data_frames] # 假设数据是随机生成的
```
6. 绘制图,横坐标为文件名,纵坐标为处理后的数据:
```python
plt.figure(figsize=(10, 6))
plt.xticks(x_values, file_names, rotation=90)
plt.plot(x_values, y_values)
plt.title('文件名对应的简单线条图')
plt.xlabel('文件名')
plt.ylabel('数据值')
plt.grid(True)
plt.show()
```
这个示例中,我们使用的是简单的线条图,实际上,横坐标应根据你的需求和文件内容来选择合适的表示形式。
相关推荐
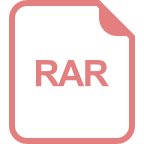
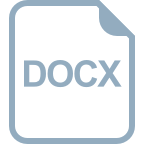
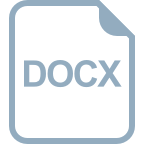
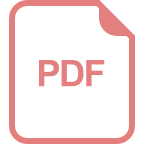
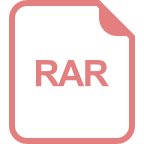
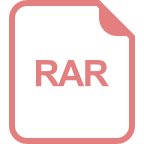
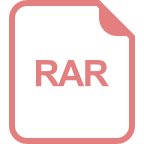
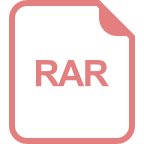
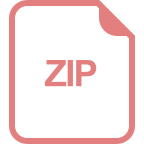
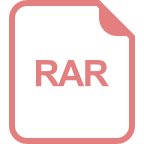
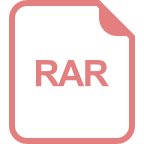
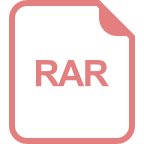
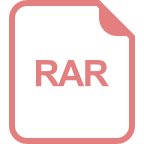
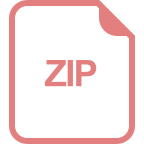
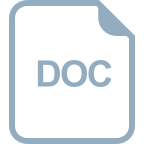
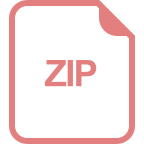
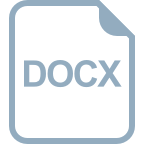