写一段python程序:文件夹G:\drought\data\data1\stationhh下保存着txt和xlsx文件,把每个表格第一行删除。
时间: 2024-09-11 16:09:25 浏览: 51
要完成这个任务,你可以使用Python的`pandas`库来处理`.xlsx`文件,以及内置的`open`函数来处理`.txt`文件。以下是一个简单的示例代码,它会遍历指定文件夹下的所有`.txt`和`.xlsx`文件,并对每个表格删除第一行。
```python
import os
import pandas as pd
from pandas import ExcelWriter
# 指定文件夹路径
folder_path = r'G:\drought\data\data1\stationhh'
# 获取文件夹下所有的.txt和.xlsx文件
files = [f for f in os.listdir(folder_path) if f.endswith(('.txt', '.xlsx'))]
# 遍历所有文件
for file in files:
file_path = os.path.join(folder_path, file)
if file.endswith('.xlsx'):
# 读取excel文件
df = pd.read_excel(file_path)
# 删除第一行
df = df.iloc[1:]
# 使用ExcelWriter写回文件
with ExcelWriter(file_path) as writer:
df.to_excel(writer, index=False)
elif file.endswith('.txt'):
# 打开并读取txt文件
with open(file_path, 'r', encoding='utf-8') as f:
lines = f.readlines()
# 删除第一行
lines = lines[1:]
# 写回文件
with open(file_path, 'w', encoding='utf-8') as f:
f.writelines(lines)
print("处理完成。")
```
请注意,这段代码假设所有的`.xlsx`文件都是Excel表格,并且没有考虑工作表(sheet)的概念。如果你的`.xlsx`文件包含多个工作表,你需要对代码进行相应的修改。同样,对于`.txt`文件,这段代码假设它们是以UTF-8编码的文本文件。
在实际使用中,请确保已经安装了`pandas`库,可以通过运行`pip install pandas`来安装。
阅读全文
相关推荐
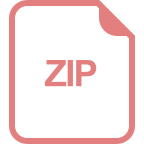
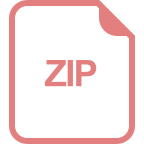
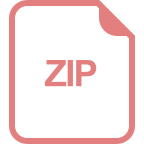














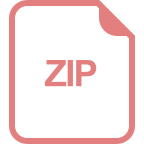