C语言如何实现某个信息查找功能
时间: 2024-10-23 17:03:33 浏览: 17
在C语言中,实现信息查找功能通常会依赖于数组、链表或哈希表等数据结构。这里以三种常见情况为例:
**1. 数组查找:**
假设有一个已排序的整数数组,我们可以使用线性搜索(遍历)的方式查找特定值:
```c
int searchArray(int arr[], int n, int target) {
for (int i = 0; i < n; i++) {
if (arr[i] == target) {
return i; // 返回目标值的索引
}
}
return -1; // 如果未找到,返回-1表示不存在
}
```
**2. 链表查找:**
对于单向链表,每个节点包含一个值和指向下一个节点的指针。查找功能同样通过遍历完成:
```c
struct Node* findInLinkedList(struct Node* head, int target) {
struct Node* current = head;
while (current != NULL && current->data != target) {
current = current->next;
}
return current;
}
```
如果找到了就返回当前节点,没找到返回NULL。
**3. 哈希表查找:**
哈希表提供了常数时间复杂度的查找能力。你可以使用预定义的哈希函数和桶数组实现:
```c
typedef struct HashNode {
int key;
int value;
struct HashNode* next;
} HashNode;
HashNode* hashTableFind(HashTable* table, int key) {
int index = hashFunction(key);
HashNode* node = table[index];
while (node != NULL) {
if (node->key == key) {
return node;
}
node = node->next;
}
return NULL;
}
```
其中`hashFunction`是自定义的哈希函数,用于计算键值对应的桶地址。
阅读全文
相关推荐
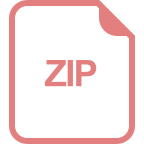
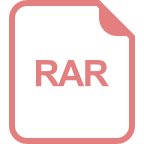
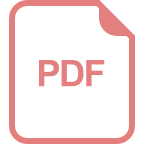
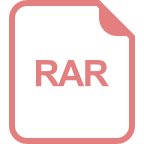
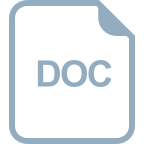
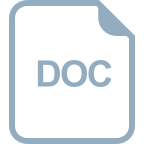
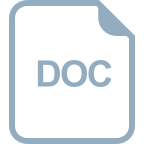
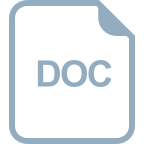
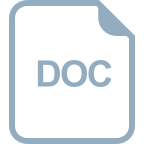
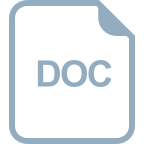
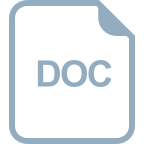
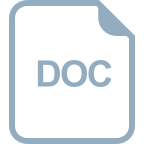

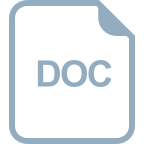
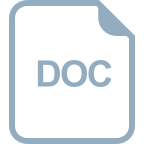
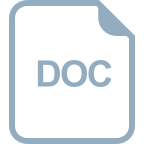
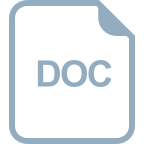
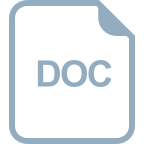
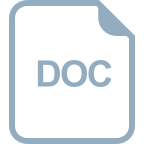