用matlab编写程序
时间: 2024-12-02 21:15:47 浏览: 6
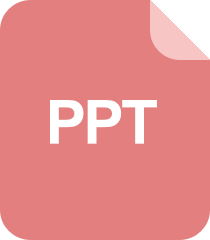
MATLAB简介及程序编写ppt课件.ppt
为了帮助您使用MATLAB编写程序来实现遗传算法(GA)和粒子群优化算法(PSO),并求解给定函数的最大值,以下是一些基本的步骤和示例代码:
### 1. 定义目标函数
假设我们需要最大化的目标函数为 \( f(x) = x \sin(10\pi x) + 2 \),其中 \( x \in [-1, 2] \)。
```matlab
function y = objective_function(x)
y = x * sin(10 * pi * x) + 2;
end
```
### 2. 遗传算法 (GA)
#### 步骤:
1. 初始化种群。
2. 计算适应度。
3. 选择操作。
4. 交叉操作。
5. 变异操作。
6. 重复上述步骤直到满足终止条件。
#### 示例代码:
```matlab
% 参数设置
pop_size = 50; % 种群大小
num_generations = 100; % 迭代次数
mutation_rate = 0.01; % 变异率
crossover_rate = 0.8; % 交叉率
lower_bound = -1; % 下界
upper_bound = 2; % 上界
% 初始化种群
population = lower_bound + (upper_bound - lower_bound) * rand(pop_size, 1);
% 主循环
for generation = 1:num_generations
% 计算适应度
fitness = arrayfun(@objective_function, population);
% 选择操作
[sorted_fitness, idx] = sort(fitness, 'descend');
sorted_population = population(idx);
% 生成新种群
new_population = zeros(size(population));
for i = 1:2:pop_size-1
parent1 = sorted_population(i);
parent2 = sorted_population(i+1);
% 交叉操作
if rand < crossover_rate
crossover_point = rand;
child1 = crossover_point * parent1 + (1 - crossover_point) * parent2;
child2 = crossover_point * parent2 + (1 - crossover_point) * parent1;
else
child1 = parent1;
child2 = parent2;
end
% 变异操作
if rand < mutation_rate
child1 = child1 + (rand - 0.5) * (upper_bound - lower_bound) / 10;
end
if rand < mutation_rate
child2 = child2 + (rand - 0.5) * (upper_bound - lower_bound) / 10;
end
new_population(i) = child1;
new_population(i+1) = child2;
end
% 替换旧种群
population = new_population;
% 输出当前最佳个体
[~, best_idx] = max(fitness);
fprintf('Generation %d: Best fitness = %.4f, Best solution = %.4f\n', generation, fitness(best_idx), population(best_idx));
end
% 最终结果
[best_fitness, best_solution_idx] = max(fitness);
fprintf('Final result: Best fitness = %.4f, Best solution = %.4f\n', best_fitness, population(best_solution_idx));
```
### 3. 粒子群优化 (PSO)
#### 步骤:
1. 初始化粒子位置和速度。
2. 计算每个粒子的适应度。
3. 更新个人最佳和全局最佳位置。
4. 更新粒子的速度和位置。
5. 重复上述步骤直到满足终止条件。
#### 示例代码:
```matlab
% 参数设置
num_particles = 50; % 粒子数量
num_iterations = 100; % 迭代次数
w = 0.7; % 惯性权重
c1 = 1.5; % 个体学习因子
c2 = 1.5; % 社会学习因子
lower_bound = -1; % 下界
upper_bound = 2; % 上界
% 初始化粒子位置和速度
positions = lower_bound + (upper_bound - lower_bound) * rand(num_particles, 1);
velocities = zeros(num_particles, 1);
personal_best_positions = positions;
personal_best_fitness = arrayfun(@objective_function, positions);
global_best_position = positions(find(personal_best_fitness == max(personal_best_fitness), 1));
% 主循环
for iteration = 1:num_iterations
% 更新速度和位置
for i = 1:num_particles
r1 = rand;
r2 = rand;
velocities(i) = w * velocities(i) + c1 * r1 * (personal_best_positions(i) - positions(i)) + c2 * r2 * (global_best_position - positions(i));
positions(i) = positions(i) + velocities(i);
% 边界处理
if positions(i) < lower_bound
positions(i) = lower_bound;
elseif positions(i) > upper_bound
positions(i) = upper_bound;
end
% 计算适应度
current_fitness = objective_function(positions(i));
% 更新个人最佳
if current_fitness > personal_best_fitness(i)
personal_best_fitness(i) = current_fitness;
personal_best_positions(i) = positions(i);
end
% 更新全局最佳
if current_fitness > objective_function(global_best_position)
global_best_position = positions(i);
end
end
% 输出当前最佳个体
fprintf('Iteration %d: Global best fitness = %.4f, Global best position = %.4f\n', iteration, objective_function(global_best_position), global_best_position);
end
% 最终结果
final_best_fitness = objective_function(global_best_position);
fprintf('Final result: Global best fitness = %.4f, Global best position = %.4f\n', final_best_fitness, global_best_position);
```
### 4. 比较两种算法
您可以运行上述两个算法,并记录每一代或每次迭代的最佳适应度和解决方案。通过绘制适应度随迭代次数的变化图,可以直观地比较两种算法的性能。
```matlab
% 绘制适应度变化图
figure;
plot(ga_best_fitness_history, 'b-', 'LineWidth', 2);
hold on;
plot(pso_best_fitness_history, 'r--', 'LineWidth', 2);
xlabel('Iteration/Generation');
ylabel('Best Fitness');
legend('Genetic Algorithm', 'Particle Swarm Optimization');
title('Comparison of GA and PSO');
grid on;
```
希望这些代码和解释对您有所帮助!如果有任何进一步的问题,请随时提问。
阅读全文
相关推荐
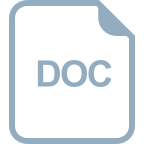
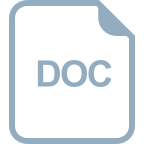
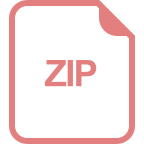
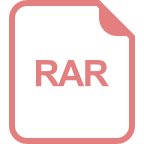
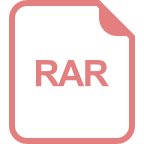
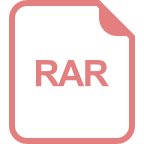
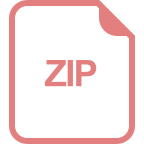
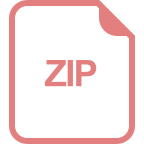
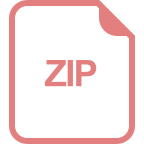