用C++语言实现一个词法分析器
时间: 2024-10-03 10:01:06 浏览: 30
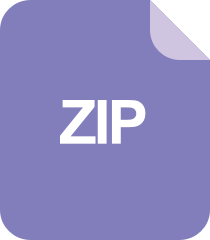
C++实现词法分析器.zip
在C++中实现词法分析器通常会利用递归下降解析(Recursive Descent Parsing),这是一种通过定义一系列函数来识别输入文本中的不同语法元素的方法。下面是一个简单的例子,展示了如何创建一个基本的词法分析器,可以识别整数、标识符和关键字:
```cpp
#include <iostream>
#include <string>
using namespace std;
enum Token {
ID, // 标识符
INTEGER, // 整数
KEYWORD // 关键字
};
// 假设我们有这样一些关键词
const string keywords[] = {"if", "else", "while", "int"};
// 定义词法单元
struct Tokenizer {
private:
string input;
int pos; // 当前字符位置
public:
Tokenizer(const string& text) : input(text), pos(0) {}
Token nextToken() {
if (pos >= input.size()) return END_TOKEN; // 如果已到结尾返回END_TOKEN
char c = input[pos];
if (isdigit(c)) { // 检查是否为数字
++pos;
string num;
while (isdigit(input[pos])) {
num += input[pos++];
}
return INTEGER, stoi(num);
} else if (isalpha(c) || c == '_') { // 检查是否为标识符或下划线开头
string id;
while (isalnum(input[pos]) || input[pos] == '_') {
id += input[pos++];
}
if (find(keywords, keywords + sizeof(keywords) / sizeof(keywords[0]), id) != end(keywords)) {
return KEYWORD, id; // 如果找到关键词
} else {
return ID, id; // 否则是标识符
}
} else { // 其他字符,如空格或标点符号
++pos;
return LITERAL, c; // 返回当前字符作为LITERAL
}
}
bool atEnd() const { return pos == input.size(); }
};
int main() {
Tokenizer tokenizer("int a = 5; if(b) { foo(); }");
while (!tokenizer.atEnd()) {
Token token = tokenizer.nextToken();
switch (token.type) {
case INTEGER:
cout << "Integer: " << token.value << endl;
break;
case ID:
cout << "Identifier: " << token.value << endl;
break;
case KEYWORD:
cout << "Keyword: " << token.value << endl;
break;
case END_TOKEN:
cout << "End of input" << endl;
break;
default:
cout << "Literal: " << static_cast<char>(token.value) << endl;
}
}
return 0;
}
```
在这个例子中,`Tokenizer`类有一个状态机性质,遍历输入字符串并根据字符的类型生成相应的令牌。`nextToken()`函数处理了各种情况,包括数字、标识符和关键字。
阅读全文
相关推荐
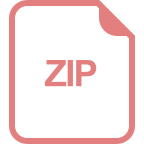
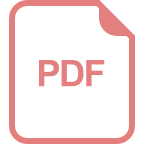
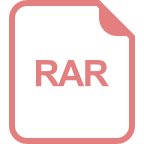
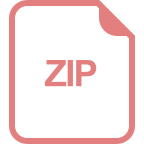

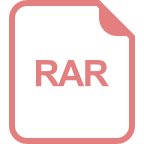
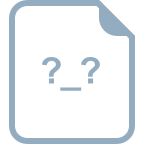
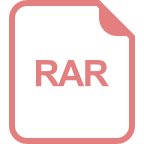
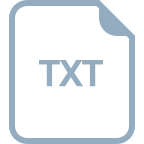
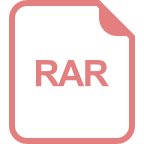
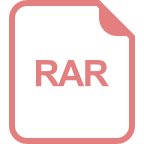
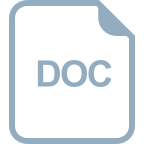
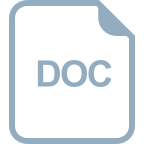


