c# CPU和内存管理
时间: 2023-11-24 18:54:33 浏览: 46
C# 是一种高级编程语言,它提供了方便的内存管理机制,称为垃圾回收(Garbage Collection)。垃圾回收器负责自动释放不再使用的内存,使开发人员无需手动管理内存。
在 C# 中,CPU 的管理主要通过编写高效的代码来实现。这包括使用适当的数据结构和算法,避免不必要的计算和循环,以及注意避免频繁的内存分配和释放。优化代码可以减少 CPU 的使用率,提高程序的性能。
对于内存管理,C# 提供了垃圾回收器来自动分配和释放内存。垃圾回收器会定期检查不再被引用的对象,并释放它们所占用的内存。这种自动化的内存管理机制减少了内存泄漏和悬挂指针等常见问题的发生。
除了垃圾回收之外,C# 还提供了一些手动管理内存的方式,如使用 `IDisposable` 接口进行资源清理和使用 `using` 语句块来确保资源被正确释放。
总结起来,C# 通过垃圾回收器实现自动的内存管理,而对于 CPU 的管理则需要开发人员编写高效的代码来提高程序性能。
相关问题
c# kernel32 cpu使用率
C是一种编程语言,其设计初衷是用于系统级的编程,由Dennis Ritchie在20世纪70年代开发。C语言是一种通用、高效、具有良好可移植性的编程语言,在软件开发中广泛应用。
C语言的优点包括:
1. 简洁高效:C语言注重性能和效率,具有高度的执行速度和较低的内存占用。
2. 可移植性:C语言编写的程序可以在不同操作系统和硬件平台上运行,具有很好的可移植性。
3. 强大的控制能力:C语言提供了丰富的控制结构和运算符,编程者可以更加灵活地控制程序的行为。
4. 大量的库支持:C语言有许多标准库和第三方库可供使用,开发者可以通过引用这些库来快速开发程序。
5. 直接访问内存:C语言提供了指针的概念,可以直接访问内存地址,方便对数据的处理和操作。
然而,C语言也存在一些缺点:
1. 相对较难学习:相比其他编程语言,C语言的语法相对较为底层和复杂,学习起来需要花费一定的时间和精力。
2. 缺乏一些现代编程特性:C语言在语言设计上相对较早,缺乏一些现代编程语言的高级特性,如面向对象编程、垃圾回收等。
3. 容易出现指针和内存错误:C语言使用指针来进行内存管理,不当的使用指针容易导致内存泄漏、越界访问等错误。
总的来说,C语言是一种功能强大、高效可移植性强的编程语言,尤其适合对性能要求较高的系统级编程和嵌入式开发。在学习和使用时,需要注意指针和内存管理等方面的细节,以避免出现错误。
C#如何读欧姆龙PLC内存区
在 C# 中,你可以使用 S7.Net 库来读取欧姆龙 PLC 的内存区。首先,你需要通过 NuGet 包管理器安装 S7.Net 库。
在你的 C# 项目中,导入 S7.Net 命名空间:
```csharp
using S7.Net;
```
然后,创建一个 S7NetPlc 对象来连接到 PLC:
```csharp
Plc plc = new Plc(CpuType.Omron, ipAddress, rack, slot);
```
其中,ipAddress 是 PLC 的 IP 地址,rack 和 slot 是 PLC 的机架号和插槽号。
接下来,你可以使用 ReadBytes 方法来读取内存区的数据。例如,如果要读取 M 区的数据,可以使用以下代码:
```csharp
byte[] data = plc.ReadBytes(DataType.DataBlock, dbNumber, offset, length);
```
其中,DataType.DataBlock 表示数据类型为数据块(DB),dbNumber 是数据块的编号,offset 是相对于数据块起始地址的偏移量,length 是要读取的字节数。
读取成功后,你可以对返回的字节数组进行解析和处理。
最后,记得在读取完成后关闭连接:
```csharp
plc.Close();
```
这是一个简单的示例来读取欧姆龙 PLC 内存区的数据。你可以根据实际情况进行适当的修改和扩展。
相关推荐
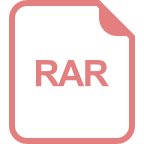












