spring boot重定向请求加入认证参数
时间: 2024-11-04 10:14:09 浏览: 57
在Spring Boot应用中,如果你想要在重定向(Redirect)请求时添加认证相关的参数,比如JWT令牌,通常会在HTTP响应头设置`Location`字段,同时将认证信息作为查询参数传递。以下是步骤:
1. **创建JWT Token**:首先确保你有一个生成JWT token的机制,例如使用Spring Security的JwtEncoder或者自定义的一个工具类。
```java
import org.springframework.security.authentication.UsernamePasswordAuthenticationToken;
import org.springframework.security.core.context.SecurityContextHolder;
import com.auth0.jwt.JWT;
import com.auth0.jwt.algorithms.Algorithm;
public String generateJwtToken(String username) {
// 使用用户名和密钥生成JWT
return JWT.create()
.withSubject(username)
.sign(Algorithm.HMAC256("your-secret-key"));
}
```
2. **登录成功后的重定向**:当用户通过身份验证并登录成功后,在`SecurityController`或其他相关控制器中处理登录,获取到JWT,然后将其添加到重定向URL的查询参数中:
```java
@GetMapping("/login")
public RedirectView login(@RequestParam String username, String password) {
// 验证用户名密码...
if (isValidUser()) {
UsernamePasswordAuthenticationToken auth = new UsernamePasswordAuthenticationToken(username, password);
SecurityContextHolder.getContext().setAuthentication(auth);
// 添加JWT到查询参数
String jwt = generateJwtToken(username);
String redirectUrl = "http://example.com/someprotectedpage?jwt=" + jwt;
return new RedirectView(redirectUrl, true); // 设置true表示永久重定向
}
// 登录失败处理...
}
```
3. **保护资源的拦截器**:在需要保护的控制器方法上,可以配置一个JWT过滤器(如JwtRequestFilter)来检查查询参数中的JWT是否有效:
```java
@Component
public class JwtRequestFilter extends OncePerRequestFilter {
@Autowired
private JwtHelper jwtHelper;
@Override
protected void doFilterInternal(HttpServletRequest request, HttpServletResponse response, FilterChain chain)
throws ServletException, IOException {
String jwt = request.getParameter("jwt");
if (jwt != null && validateJwt(jwt)) {
// JWT有效,继续执行后续操作
chain.doFilter(request, response);
} else {
// JWT无效,处理未授权或返回错误页面
response.sendRedirect("/unauthorized"); // 或者返回401状态码
}
}
private boolean validateJwt(String jwt) {
try {
JWT.verify(jwt, Algorithm.HMAC256("your-secret-key"));
return true;
} catch (Exception e) {
return false;
}
}
}
```
阅读全文
相关推荐
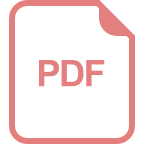
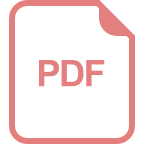
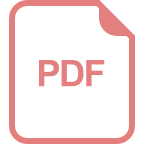















