练习python中的封装的使用
时间: 2024-10-17 17:10:19 浏览: 23
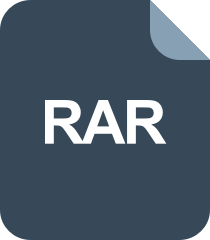
python中基础知识和练习
在Python中,封装是一种重要的面向对象编程特性,它允许我们将数据和操作这些数据的方法组合在一起形成一个独立的对象。以下是封装的一些关键点:
1. **定义类**: 使用`class`关键字创建一个类,它是封装的起点。类定义了一个对象的数据(属性)和行为(方法)。
```python
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
```
这里,`name`和`age`是私有变量(也称为实例变量),它们被`self.`前缀标识,外部无法直接访问。
2. **封装属性**: 使用`__get__` 和 `__set__`特殊方法(也称作魔术方法)可以控制属性的读取和修改,提供验证机制。
```python
class SecurePerson(Person):
def __getattribute__(self, item):
if item == 'age':
return super().__getattribute__('age')
raise AttributeError(f"{item} is not accessible")
def set_age(self, age):
if 0 < age <= 150:
super().set_age(age)
else:
print("Age must be between 0 and 150.")
```
在这个例子中,年龄属性只能在特定范围内设置,并隐藏了具体的年龄值。
3. **公共接口**: 需要暴露给用户使用的部分通常通过公共方法(public methods)完成,如获取信息或更改状态。
```python
def introduce_yourself(self):
print(f"My name is {self.name}, I am {self.age} years old.")
person = SecurePerson("Alice", 30)
person.introduce_yourself()
```
阅读全文
相关推荐
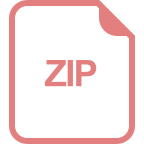
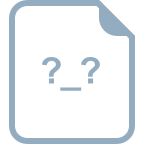
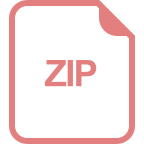
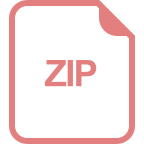
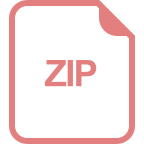
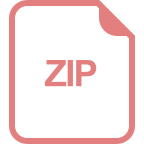
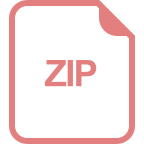
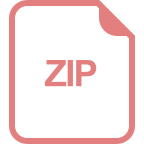
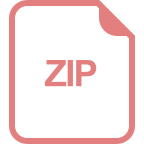
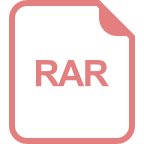
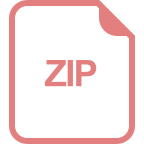
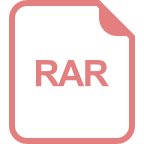
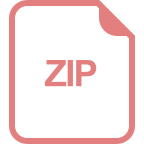
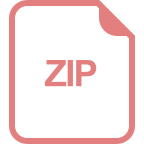
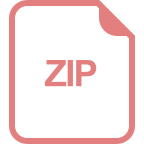
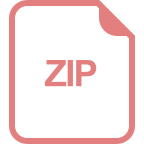