python使用队列实现凯撒加密
时间: 2024-09-22 17:08:56 浏览: 71
在Python中,可以使用内置的`collections`模块中的`deque`(双端队列)来实现凯撒密码的简单版本,因为凯撒加密本质上是对字符进行位移操作,而`deque`提供了一个方便的添加和删除元素的机制。以下是使用`deque`实现凯撒加密的一个简短示例:
```python
from collections import deque
def caesar_cipher_encrypt(text, shift):
encrypted_text = []
# 使用deque存储字符并进行位移操作
char_queue = deque(text)
for _ in range(len(char_queue)):
if char_queue[0].isalpha(): # 检查是否为字母
shifted_char = chr(((ord(char_queue[0]) - ord('a') + shift) % 26) + ord('a')) if char_queue[0].islower() else chr(((ord(char_queue[0]) - ord('A') + shift) % 26) + ord('A'))
else:
shifted_char = char_queue[0] # 非字母字符不变
encrypted_text.append(shifted_char)
char_queue.rotate(-1) # 移除第一个字符并添加到队尾
return ''.join(encrypted_text)
# 示例
plaintext = "Hello, World!"
shift = 3
encrypted = caesar_cipher_encrypt(plaintext, shift)
print(f"原始文本: {plaintext}")
print(f"加密后的文本: {encrypted}")
阅读全文
相关推荐
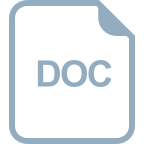
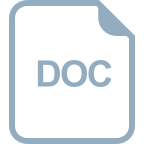
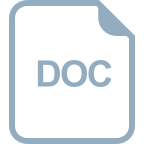
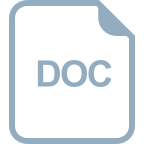
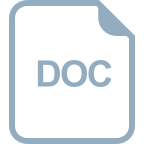
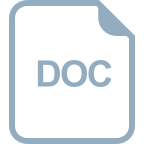
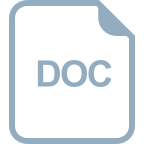
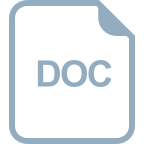
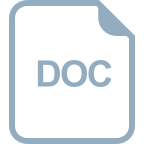
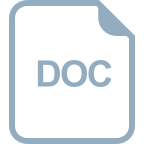
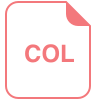

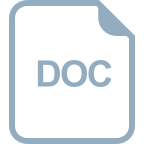
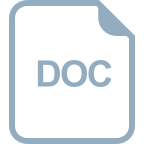
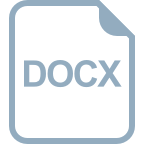
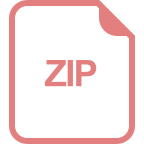