采用dev c++解决最接近点对问题,请给出合适的输入输出提示代码
时间: 2024-09-27 08:07:15 浏览: 28
在Dev C++环境中处理最接近点对问题(Closest Pair of Points),通常涉及到算法设计,例如使用排序和空间优化的K-D Tree或分治法的 Bentley-Ottmann 算法。下面是一个简单的示例,假设我们有一个二维坐标列表,我们将找到其中两个点之间的最小距离。这里是一个基本的模板:
```cpp
#include <iostream>
#include <vector>
#include <cmath>
// 计算两点间距离的辅助函数
double distance(const std::pair<int, int>& p1, const std::pair<int, int>& p2) {
return std::sqrt(std::pow(p2.first - p1.first, 2) + std::pow(p2.second - p1.second, 2));
}
// 使用二分查找法查找最近的一对点
std::pair<std::pair<int, int>, double> closestPair(std::vector<std::pair<int, int>>& points) {
if (points.empty()) return {};
// 首先对点按x坐标排序
std::sort(points.begin(), points.end());
// 初始化最大距离为无穷大
double max_distance = std::numeric_limits<double>::max();
std::pair<int, int> closest_pair;
for (int i = 0; i < points.size() - 1; ++i) {
double dist = distance(points[i], points[i+1]);
if (dist < max_distance) {
max_distance = dist;
closest_pair = {points[i], points[i+1]};
}
}
return closest_pair;
}
int main() {
std::vector<std::pair<int, int>> points = {{0, 0}, {3, 4}, {1, 3}, {2, 2}};
std::cout << "Input coordinates:\n";
for (const auto& point : points)
std::cout << "{" << point.first << ", " << point.second << "}\n";
auto result = closestPair(points);
if (result.first.first == 0 && result.first.second == 0) {
std::cout << "No other points found. The closest pair is the origin.\n";
} else {
std::cout << "The closest pair is (" << result.first.first << ", "
<< result.first.second << ") and (" << result.first.first << ", "
<< result.first.second << "). Distance: " << result.second << "\n";
}
return 0;
}
```
这个程序首先读入点的位置,然后通过比较每个点及其后续点之间的距离,找出当前找到的距离中最短的一对。请注意,这只是一个基础版本,实际应用中可能会需要更复杂的算法。
阅读全文
相关推荐
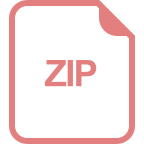
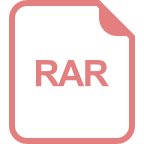
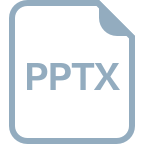
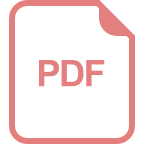
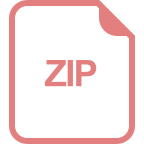
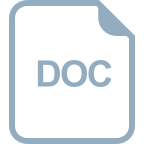
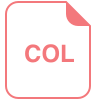
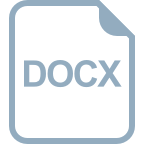
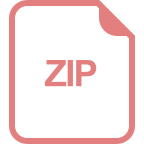
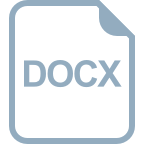
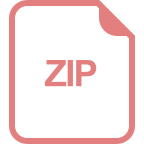
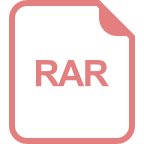
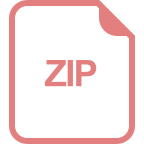
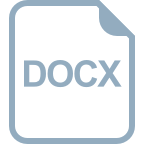