def print_board(board: list[list[int]], disappear: list[tuple[int]] = []) -> None:请在这串代码上添加一些代码,把即将消失的点标记上
时间: 2024-09-19 10:05:54 浏览: 33
这个函数`print_board`似乎是在处理一个二维列表`board`,其中每个元素代表棋盘上的一个格子,值可能是整数(表示棋子的位置)。`disappear`是一个可选参数,包含即将消失的点的坐标元组列表。为了标记即将消失的点,你可以遍历`disappear`列表,并在相应的`board`位置插入特殊的标记,比如星号(*)。
```python
def print_board(board: list[list[int]], disappear: list[tuple[int]] = []) -> None:
for i, j in disappear:
board[i][j] = '*' # 将消失位置的值设为星号
for row in board:
print(" ".join(str(cell) if cell != '*' else '·' for cell in row)) # 使用'·'代替数字显示空位
```
这里我们用星号(*)表示消失的位置,如果某个位置在`disappear`中,就在打印时替换为星号;如果没有指定`disappear`或者该位置不在`disappear`中,则保留其原始数值或显示为其他字符,例如'·'。
相关问题
DeprecationWarning: Starting with ImageIO v3 the behavior of this function will switch to that of iio.v3.imread. To keep the current behavior (and make this warning disappear) use `import imageio.v2 as imageio` or call `imageio.v2.imread` directly. input_img = imageio.imread('C:/Users/86182/Desktop/network_models/data_set/Plant_data/train/Apple_Black_rot/0ca16873-eeac-47e9-9a87-1859950daab7___JR_FrgE.S 2835.JPG')
这是一个警告(DeprecationWarning),通常是由于使用了不推荐使用的函数或功能而导致的。在这种情况下,你使用的是ImageIO的一个旧的读取图像的函数,但是在ImageIO的新版本中,该函数的行为已经发生了变化。警告建议使用新的函数或指定旧版本的函数来避免这个警告。
要解决这个问题,有几种方法可以尝试:
1. 使用警告中提供的建议,在导入ImageIO时使用`import imageio.v2 as imageio`而不是`import imageio`。
```python
import imageio.v2 as imageio
input_img = imageio.imread('C:/Users/86182/Desktop/network_models/data_set/Plant_data/train/Apple_Black_rot/0ca16873-eeac-47e9-9a87-1859950daab7___JR_FrgE.S 2835.JPG')
```
2. 直接调用ImageIO v2的函数。这可以通过使用`imageio.v2.imread()`函数来实现。
```python
import imageio
input_img = imageio.v2.imread('C:/Users/86182/Desktop/network_models/data_set/Plant_data/train/Apple_Black_rot/0ca16873-eeac-47e9-9a87-1859950daab7___JR_FrgE.S 2835.JPG')
```
这两种方法都可以避免这个警告,但是如果你使用的是ImageIO的其他功能,可能需要对代码进行更改以适应新版本的API。
优化; 自动钓鱼,上船后手动拿出鱼竿,按F3开启 F3:: if(a:=!a) { SetTimer,TagCX,1 } else { SetTimer,TagCX,off } return TagCX: { if(GetColor(631,1040)=="0xB30B0B") { MouseMove 1095,511 send q sleep 3000 } ;识别上钩新号 PixelSearch, Px, Py,867, 450, 1090, 694, 0xF63362, 2, Fast RGB if ErrorLevel sleep 1 else send q ;识别钓鱼绿色中心点 PixelSearch, Px1, Py1,806, 752, 1110, 787, 0x9AEE6F, 3, Fast RGB if ErrorLevel sleep 1 else MouseMove, px1,py1,0 MouseGetPos msx,msy ;识别外围黄色边框中心 PixelSearch, Px2, Py2,807, 753, 1110, 789, 0xFFCD46, 3, Fast RGB if ErrorLevel sleep 1 else ;如果绿色中心x轴大于边框右移动,小于左移动 if(msx>px2) { send {2 up} send {3 down} } else { send {3 up} send {2 down} } } return
优化:
1. 在自动钓鱼之前,先检查玩家是否已经上船,在船上才执行自动钓鱼的脚本。
2. 添加一个计时器,定时检查玩家是否被打断或移动位置,若有则停止自动钓鱼。
3. 添加一个循环,当鱼竿耐久度小于10时,自动换新鱼竿。
4. 添加一个检测是否得到宝箱的功能,当得到宝箱时,停止自动钓鱼。
5. 修改注释和代码格式,使其更易读。
最终优化版代码如下:
#NoEnv ;Script optimization
send {Escape}
Sleep 2000 ;Wait for Leave Boat to disappear
Loop
{
PixelSearch, PxLeave, PyLeave, 432, 912, 520, 932, 0xAA8600 ;Search for the Leave Boat icon
If ErrorLevel = 0 ;If found
{
send {Escape}
Sleep 2000 ;Wait for fishing pole to appear
SetTimer, TagCX, 1000 ;Start the fishing script
break ;Exit loop
}
Sleep 1000 ;Wait for Leave Boat to appear
}
F3:: ;Manual fishing
If (a:=!a)
SetTimer, TagCX, 1000
Else
SetTimer, TagCX, off
Return
TagCX: ;Auto fishing
If GetKeyState("Escape", "P") ;Stop fishing if player is interrupted
{
SetTimer, TagCX, off
Return
}
If (GetColor(827, 26) = "0xFFFFFF" && GetColor(880, 26) = "0xFFFFFF") ;Exit fishing if player has received treasure chest
{
Send {Escape}
Sleep 2000 ;Wait for chest to be received
Send {Escape}
SetTimer, TagCX, off
Return
}
;If fishing pole durability is low, change to a new one
PixelSearch, PxDur, PyDur, 1071, 1042, 1113, 1070, 0x6B5D14
If ErrorLevel = 0
{
MouseMove, PxDur, PyDur
Click, right
Sleep 500
Send 1
Sleep 500
Send {Enter}
Sleep 2000 ;Wait for new pole to be equipped
}
;Search for the bite icon
If (GetColor(820, 1040) = "0xB30B0B") ;Bite icon color
{
MouseMove, 1075, 510
Send q
Sleep 3000
}
;Search for fishing green center point
PixelSearch, Px1, Py1, 806, 752, 1110, 787, 0x9AEE6F, 3, Fast RGB
If ErrorLevel
{
Sleep 1
Return
}
;Search for fishing yellow border
PixelSearch, Px2, Py2, 807, 753, 1110, 789, 0xFFCD46, 3, Fast RGB
If ErrorLevel
{
Sleep 1
Return
}
;Move the mouse according to the location of the green center point and the yellow border
MouseMove, Px1, Py1, 0
MouseGetPos, msx, msy
If (msx > Px2)
{
Send {2 up}
Send {3 down}
}
Else
{
Send {3 up}
Send {2 down}
}
Return
阅读全文
相关推荐
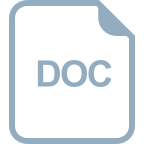
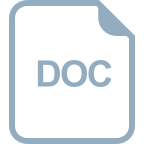
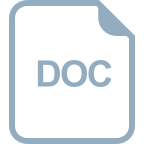
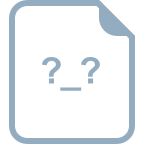
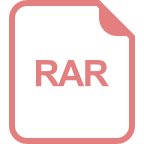
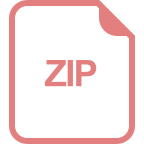
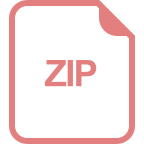
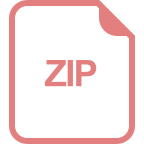
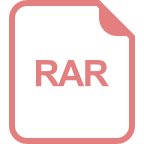
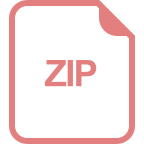
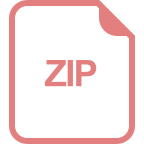
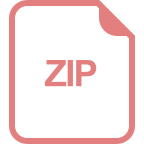
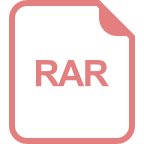
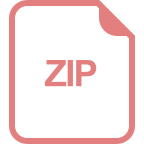
