用HB表格加按钮做名茶馆茶叶订购的代码
时间: 2024-12-09 11:29:16 浏览: 8
在HTML和JavaScript中,你可以创建一个简单的茶叶订购表单,结合Bootstrap表格(HB表格)和按钮功能。这里是一个基础示例:
```html
<!DOCTYPE html>
<html lang="zh">
<head>
<meta charset="UTF-8">
<title>茶叶订购</title>
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css">
</head>
<body>
<div class="container mt-5">
<table class="table table-bordered">
<thead>
<tr>
<th>茶叶名称</th>
<th>单价(元)</th>
<th>数量</th>
<th>操作</th>
</tr>
</thead>
<tbody id="tea-table-body">
<!-- 使用JavaScript动态填充茶叶信息 -->
</tbody>
</table>
<div class="form-group mt-3">
<label for="newTea">添加新茶叶:</label>
<input type="text" class="form-control" id="newTea" placeholder="茶叶名称">
<input type="number" class="form-control" id="newPrice" placeholder="单价(元)">
</div>
<button type="button" class="btn btn-primary" onclick="addTea()">添加</button>
</div>
<script>
function addTea() {
const newTea = document.getElementById("newTea").value;
const newPrice = document.getElementById("newPrice").value;
if (newTea && newPrice) {
const newRow = `<tr>
<td>${newTea}</td>
<td>${newPrice}</td>
<td><input type="number" class="form-control"></td>
<td><button class="btn btn-danger ml-2" onclick="removeRow(this)">删除</button></td>
</tr>`;
document.getElementById("tea-table-body").innerHTML += newRow;
}
}
function removeRow(button) {
button.parentElement.remove();
}
// 初始化示例数据
addTea(); // 需要在实际项目中动态生成数据
</script>
</body>
</html>
```
在这个例子中,用户可以输入新的茶叶名称和单价,点击“添加”按钮会将新行添加到表格中。每个新行还有删除按钮,点击时会通过JavaScript删除对应的行。
阅读全文
相关推荐
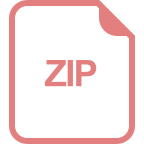
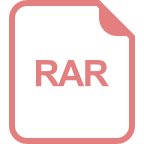
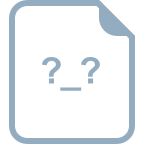

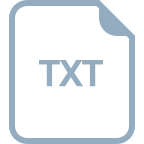
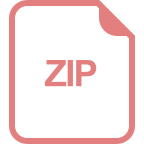
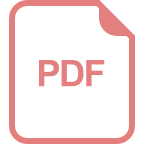
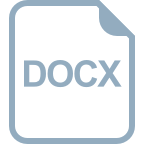
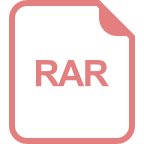
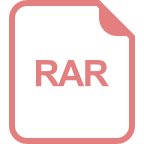
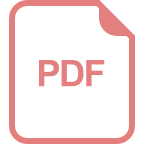
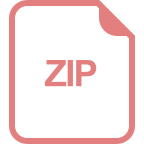
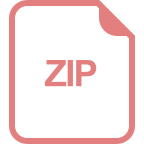
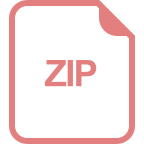
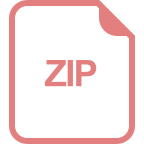
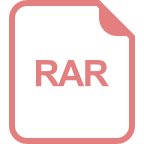
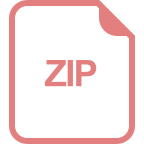