用java写一个桌面程序,能从文件选择框选择文件,让打印机来打印
时间: 2024-10-18 13:11:24 浏览: 18
在Java中创建一个简单的桌面应用程序,让用户从文件选择框选取文件并打印出来,可以使用Swing库来构建图形用户界面,以及Java PrintService API来控制打印机。以下是简化的步骤:
```java
import javax.swing.*;
import java.awt.*;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.awt.print.Printable;
import java.awt.print.PrinterException;
public class FilePrintExample extends JFrame {
private JButton browseButton;
private JLabel filePathLabel;
private FileInputStream fileInputStream;
public FilePrintExample() {
// 初始化窗口组件
setTitle("File Print Example");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(400, 150);
JPanel panel = new JPanel(new FlowLayout());
filePathLabel = new JLabel("No file selected");
panel.add(filePathLabel);
browseButton = new JButton("Browse...");
browseButton.addActionListener(e -> selectFile());
panel.add(browseButton);
add(panel);
setVisible(true);
}
private void selectFile() {
JFileChooser chooser = new JFileChooser();
int result = chooser.showOpenDialog(this);
if (result == JFileChooser.APPROVE_OPTION) {
File selectedFile = chooser.getSelectedFile();
try {
fileInputStream = new FileInputStream(selectedFile);
filePathLabel.setText(selectedFile.getAbsolutePath());
printFile();
} catch (IOException ex) {
JOptionPane.showMessageDialog(this, "Error opening file", "Error", JOptionPane.ERROR_MESSAGE);
}
}
}
private void printFile() {
PrinterJob printerJob = PrinterJob.getPrinterJob();
try {
Printable printable = new Printable() {
@Override
public int print(Graphics graphics, PageFormat pageFormat, int pageIndex) throws PrinterException {
if (pageIndex > 0) {
return Printable.NO_SUCH_PAGE;
}
graphics.drawImage(ImageIO.read(fileInputStream), 0, 0, null);
return Printable.PAGE_EXISTS;
}
};
if (!printerJob.print(printable)) {
System.out.println("Failed to print the file.");
} else {
System.out.println("File successfully printed.");
}
} catch (IOException | PrinterException e) {
JOptionPane.showMessageDialog(this, "Error printing file", "Error", JOptionPane.ERROR_MESSAGE);
} finally {
if (fileInputStream != null) {
try {
fileInputStream.close();
} catch (IOException ex) {
ex.printStackTrace();
}
}
}
}
public static void main(String[] args) {
EventQueue.invokeLater(FilePrintExample::new);
}
}
```
这个例子中,我们创建了一个`JFrame`,包含一个文件选择按钮和一个标签显示选中的文件路径。当用户点击“浏览”按钮后,会打开文件选择对话框,选择文件后,读取文件内容并通过`Printable`接口将图片数据传递给打印机。
阅读全文
相关推荐



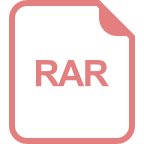
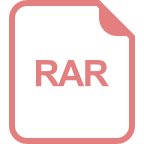
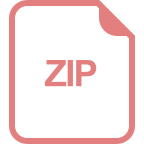


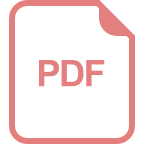

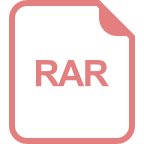
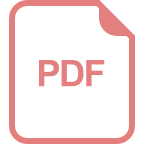






