elementui点击按钮跳转页面
时间: 2023-09-07 21:11:20 浏览: 73
您可以使用 Element UI 提供的按钮组件,并结合路由功能来实现点击按钮跳转页面的功能。
首先,确保您已经正确引入了 Element UI 组件库和 Vue Router。
然后,在您的 Vue 组件中,可以按照以下步骤来实现点击按钮跳转页面的功能:
1. 在模板中使用 Element UI 的按钮组件,并为按钮添加点击事件处理函数。例如:
```html
<template>
<div>
<el-button @click="redirectToOtherPage">跳转页面</el-button>
</div>
</template>
```
2. 在组件的 methods 中定义 redirectToOtherPage 方法,该方法将会在按钮被点击时被调用。在该方法中,使用 Vue Router 的编程式导航来实现页面跳转。例如:
```javascript
<script>
export default {
methods: {
redirectToOtherPage() {
// 使用 this.$router.push 方法进行页面跳转
this.$router.push('/other-page') // 替换为你想要跳转的页面路径
}
}
}
</script>
```
这样,当用户点击按钮时,页面将会跳转到指定的页面。
请注意,以上代码只是一个示例,实际应用中需要根据您的路由配置和项目结构进行相应的调整。
相关问题
elementui实现按钮跳转页面功能
要在 Element UI 中实现按钮跳转页面的功能,你可以使用 `<el-button>` 组件,并结合路由功能来实现。下面是一个示例:
1. 首先,确保你已经安装了 Vue Router 和 Element UI。
2. 在你的 Vue 组件中,引入所需的库和组件:
```javascript
import Vue from 'vue'
import Router from 'vue-router'
import ElementUI from 'element-ui'
import 'element-ui/lib/theme-chalk/index.css'
Vue.use(Router)
Vue.use(ElementUI)
```
3. 在 Vue Router 的配置文件中,定义你的路由:
```javascript
import Vue from 'vue'
import Router from 'vue-router'
import HomePage from '@/components/HomePage.vue'
import AboutPage from '@/components/AboutPage.vue'
Vue.use(Router)
export default new Router({
routes: [
{
path: '/',
name: 'Home',
component: HomePage
},
{
path: '/about',
name: 'About',
component: AboutPage
}
]
})
```
4. 在你的页面组件中,使用 `<el-button>` 组件,并通过 `this.$router.push()` 方法来实现页面跳转:
```html
<template>
<div>
<el-button type="primary" @click="goToHomePage">Go to Home</el-button>
<el-button type="primary" @click="goToAboutPage">Go to About</el-button>
</div>
</template>
<script>
export default {
methods: {
goToHomePage() {
this.$router.push('/')
},
goToAboutPage() {
this.$router.push('/about')
}
}
}
</script>
```
在上述示例中,我们定义了两个按钮,分别触发 `goToHomePage` 和 `goToAboutPage` 方法,在方法中使用 `this.$router.push()` 方法进行路由跳转。
这样,当你点击这些按钮时,页面就会根据定义的路由配置进行跳转。
希望这个示例能帮助到你实现按钮跳转页面的功能!
vue2+elementui点击详情通过id跳转详情页面
假设你的列表页是一个 Vue 组件,里面有一个表格用来展示数据,每一行数据都有一个 id 字段,点击某一行数据的时候需要跳转到详情页并且将对应的 id 传递过去。
可以通过以下步骤实现:
1. 在表格中添加一个“详情”按钮或者使用 element-ui 的 table-column 组件的 slot-scope 插槽来自定义一列。
```
<el-table :data="tableData">
<el-table-column label="标题">
<template slot-scope="scope">
{{ scope.row.title }}
</template>
</el-table-column>
<el-table-column>
<template slot-scope="scope">
<el-button type="text" @click="goDetail(scope.row.id)">详情</el-button>
</template>
</el-table-column>
</el-table>
```
2. 在组件的 methods 中添加 goDetail 方法。
```
methods: {
goDetail(id) {
this.$router.push(`/detail/${id}`)
}
}
```
3. 在路由配置中添加详情页的路由。
```
{
path: '/detail/:id',
name: 'Detail',
component: Detail
}
```
4. 在详情页组件中通过 $route.params.id 获取传递过来的 id。
```
<template>
<div>
<h1>{{ id }}</h1>
<!-- 其他详情内容 -->
</div>
</template>
<script>
export default {
name: 'Detail',
computed: {
id() {
return this.$route.params.id
}
}
}
</script>
```
相关推荐
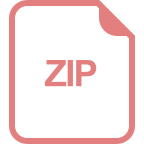
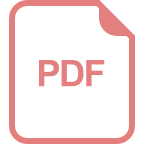
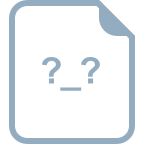












