AES加密算法java代码
时间: 2024-07-30 16:00:22 浏览: 55
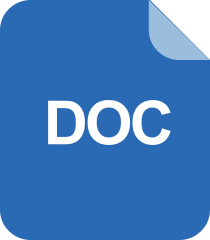
JAVA实现AES加密算法代码.doc
AES(Advanced Encryption Standard)是一种高级加密标准,用于对数据进行块级加密。Java中使用`javax.crypto.Cipher`类和`javax.crypto.spec.SecretKeySpec`来实现AES加密。以下是一个简单的AES加解密示例:
```java
import javax.crypto.Cipher;
import javax.crypto.spec.SecretKeySpec;
import java.security.Key;
public class AESExample {
private static final String ALGORITHM = "AES";
private static final byte[] keyValue = "YourSecretKey1234567890".getBytes(); // 用你的密钥替换
public static String encrypt(String value) throws Exception {
Key secretKey = generateSecretKey();
Cipher cipher = Cipher.getInstance(ALGORITHM);
cipher.init(Cipher.ENCRYPT_MODE, secretKey);
byte[] encryptedValue = cipher.doFinal(value.getBytes());
return bytesToHex(encryptedValue);
}
public static String decrypt(String encryptedValue) throws Exception {
Key secretKey = generateSecretKey();
Cipher cipher = Cipher.getInstance(ALGORITHM);
cipher.init(Cipher.DECRYPT_MODE, secretKey);
byte[] decryptedValue = cipher.doFinal(hexToBytes(encryptedValue));
return new String(decryptedValue);
}
private static Key generateSecretKey() throws Exception {
SecretKeySpec secretKeySpec = new SecretKeySpec(keyValue, ALGORITHM);
return secretKeySpec;
}
private static byte[] bytesToHex(byte[] bytes) {
StringBuilder result = new StringBuilder();
for (byte b : bytes) {
result.append(String.format("%02x", b));
}
return result.toString().getBytes();
}
private static byte[] hexToBytes(String hexString) {
int len = hexString.length();
byte[] data = new byte[len / 2];
for (int i = 0; i < len; i += 2) {
data[i / 2] = (byte) ((Character.digit(hexString.charAt(i), 16) << 4)
+ Character.digit(hexString.charAt(i+1), 16));
}
return data;
}
}
```
在这个例子中,你需要提供一个密钥字符串(替换`"YourSecretKey1234567890"`),`encrypt`方法用于加密,`decrypt`方法用于解密。
阅读全文
相关推荐
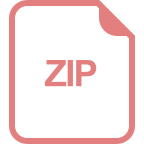
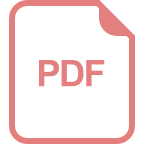
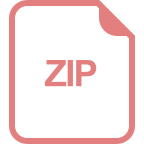
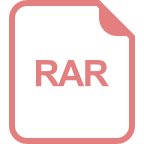
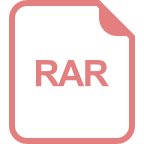
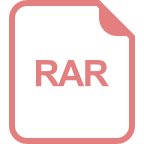
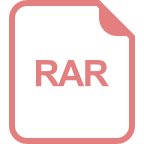
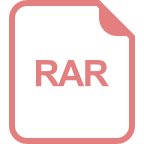
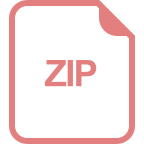







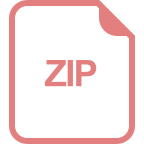