友盟推送集成springboot
时间: 2023-11-20 09:57:07 浏览: 511
友盟推送集成Spring Boot可以通过友盟提供的Java SDK来实现。具体步骤如下:
1.在pom.xml文件中添加友盟推送的Java SDK依赖:
```xml
<dependency>
<groupId>com.umeng</groupId>
<artifactId>umeng-message</artifactId>
<version>1.3.2</version>
</dependency>
```
2.在application.properties文件中添加友盟推送的配置信息:
```properties
# 友盟推送配置
umeng.appkey=your_appkey
umeng.appMasterSecret=your_app_master_secret
umeng.productionMode=false
```
3.编写友盟推送的Java代码:
```java
import com.alibaba.fastjson.JSONObject;
import com.umeng.message.*;
import com.umeng.message.common.inter.ITagManager;
import com.umeng.message.entity.UMessage;
import com.umeng.message.tag.TagManager;
import java.util.List;
public class UmengPushService implements UmengNotificationService {
private final AndroidNotification androidNotification;
private final IOSNotification iosNotification;
private final PushClient client;
public UmengPushService(String appKey, String appMasterSecret, boolean productionMode) {
androidNotification = new AndroidNotification();
iosNotification = new IOSNotification();
client = new PushClient();
client.setAppKey(appKey);
client.setAppMasterSecret(appMasterSecret);
client.setProductionMode(productionMode);
}
@Override
public void sendUnicast(String deviceToken, String title, String text, JSONObject extra) throws Exception {
UMessage message = new UMessage();
message.setDeviceToken(deviceToken);
message.setTitle(title);
message.setText(text);
message.setExtra(extra);
androidNotification.setAlert(text);
iosNotification.setAlert(text);
message.setNotificationAndroid(androidNotification);
message.setNotificationIOS(iosNotification);
client.send(message);
}
@Override
public void sendListcast(List<String> deviceTokens, String title, String text, JSONObject extra) throws Exception {
UMessage message = new UMessage();
message.setDeviceToken(deviceTokens);
message.setTitle(title);
message.setText(text);
message.setExtra(extra);
androidNotification.setAlert(text);
iosNotification.setAlert(text);
message.setNotificationAndroid(androidNotification);
message.setNotificationIOS(iosNotification);
client.send(message);
}
@Override
public void sendBroadcast(String title, String text, JSONObject extra) throws Exception {
UMessage message = new UMessage();
message.setTitle(title);
message.setText(text);
message.setExtra(extra);
androidNotification.setAlert(text);
iosNotification.setAlert(text);
message.setNotificationAndroid(androidNotification);
message.setNotificationIOS(iosNotification);
client.send(message);
}
@Override
public void addTag(String deviceToken, String tag) throws Exception {
ITagManager tagManager = new TagManager();
tagManager.add(deviceToken, tag);
}
@Override
public void deleteTag(String deviceToken, String tag) throws Exception {
ITagManager tagManager = new TagManager();
tagManager.delete(deviceToken, tag);
}
}
```
4.在Spring Boot中使用友盟推送:
```java
@RestController
@RequestMapping("/push")
public class PushController {
@Autowired
private UmengNotificationService umengNotificationService;
@PostMapping("/unicast")
public void unicast(@RequestParam String deviceToken, @RequestParam String title, @RequestParam String text, @RequestParam JSONObject extra) throws Exception {
umengNotificationService.sendUnicast(deviceToken, title, text, extra);
}
@PostMapping("/listcast")
public void listcast(@RequestParam List<String> deviceTokens, @RequestParam String title, @RequestParam String text, @RequestParam JSONObject extra) throws Exception {
umengNotificationService.sendListcast(deviceTokens, title, text, extra);
}
@PostMapping("/broadcast")
public void broadcast(@RequestParam String title, @RequestParam String text, @RequestParam JSONObject extra) throws Exception {
umengNotificationService.sendBroadcast(title, text, extra);
}
@PostMapping("/addTag")
public void addTag(@RequestParam String deviceToken, @RequestParam String tag) throws Exception {
umengNotificationService.addTag(deviceToken, tag);
}
@PostMapping("/deleteTag")
public void deleteTag(@RequestParam String deviceToken, @RequestParam String tag) throws Exception {
umengNotificationService.deleteTag(deviceToken, tag);
}
}
```
以上代码演示了如何在Spring Boot中使用友盟推送,包括单播、列播、广播和添加/删除标签等操作。需要注意的是,以上代码仅供参考,具体实现需要根据自己的业务需求进行调整。
阅读全文
相关推荐
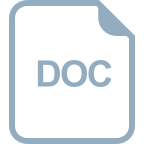
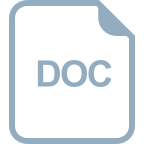
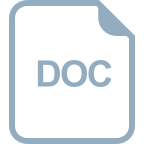
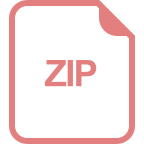
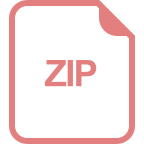
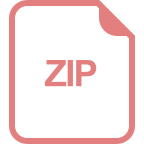
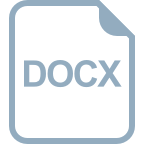
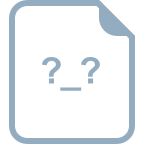
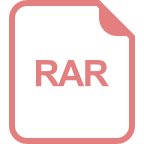
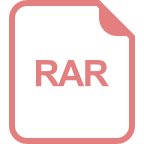
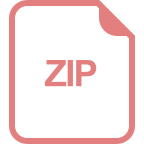
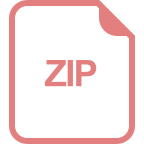
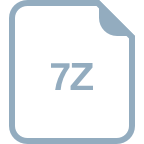
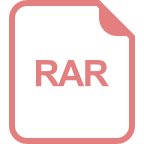
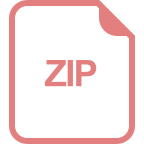
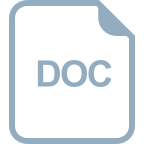

