根据所给的一段黑神话的文本,构建单词表和出现次数的字典。 3、输出频率最高的10个单词,去除冠词、代词和介词等无意义的单词。 4、可以定义多个函数分别实现独立的功能。在主模块中串联各个函数,实现总体上的功能
时间: 2024-09-12 17:07:31 浏览: 70
要根据给定的文本构建单词表并计算单词出现的频率,我们可以按照以下步骤进行:
1. 预处理文本:首先,我们需要对文本进行预处理,去除标点符号、数字,并将所有单词转换为小写,以便统计时不区分大小写。
2. 分词:将预处理后的文本分割成单词。
3. 构建单词表:创建一个字典来存储单词及其出现的次数。
4. 过滤无意义单词:创建一个列表包含所有需要被过滤的词性,如冠词、代词和介词等。
5. 统计频率:遍历分词后的单词,更新单词表中单词的计数,并过滤掉无意义的单词。
6. 输出前10个频率最高的单词:根据单词表中的计数,选出出现次数最多的10个单词。
下面是这个过程的伪代码实现:
```python
import string
def preprocess_text(text):
# 移除标点符号
text = text.translate(str.maketrans('', '', string.punctuation))
# 移除数字
text = ''.join(filter(lambda x: not x.isdigit(), text))
# 转换为小写
text = text.lower()
return text
def tokenize(text):
# 分词,这里简单使用空格分割
return text.split()
def build_word_frequency_table(tokens):
# 创建单词频率字典
frequency_table = {}
# 遍历分词结果
for token in tokens:
# 如果单词不在过滤列表中,则计数
if token not in stopwords:
frequency_table[token] = frequency_table.get(token, 0) + 1
return frequency_table
def get_top_n_frequent_words(frequency_table, n=10):
# 根据计数获取前N个最频繁出现的单词
return sorted(frequency_table.items(), key=lambda x: x[1], reverse=True)[:n]
# 主模块
def main():
text = "给定的黑神话文本内容"
# 预处理文本
preprocessed_text = preprocess_text(text)
# 分词
tokens = tokenize(preprocessed_text)
# 构建单词频率表
word_frequency_table = build_word_frequency_table(tokens)
# 获取前10个最频繁出现的单词
top_10_words = get_top_n_frequent_words(word_frequency_table)
# 输出结果
for word, count in top_10_words:
print(f"单词: {word}, 出现次数: {count}")
# 停用词列表,这里应根据实际情况填充
stopwords = set([
'the', 'a', 'an', 'and', 'or', 'but', 'is', 'are', 'of', 'to', 'in', 'for', 'on', 'with', 'as', 'by', 'that', 'this', 'it'
])
if __name__ == "__main__":
main()
```
阅读全文
相关推荐
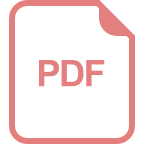
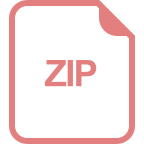
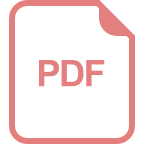
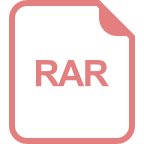
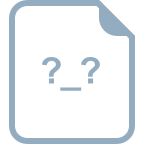
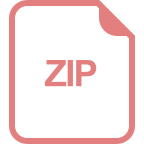
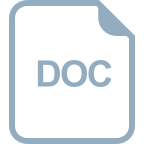
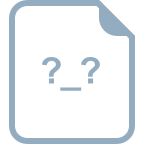
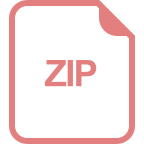
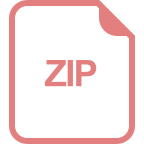
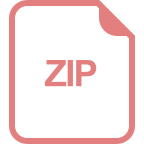
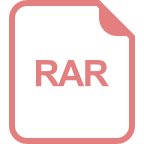
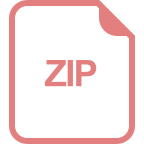
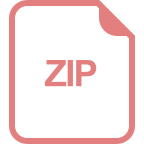
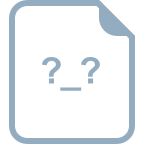
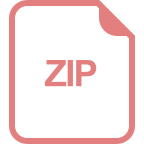
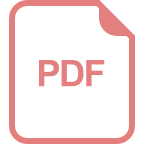
