对于一个C语言的加密算法,我需要使用wpf生成一个窗口,运用该算法对得到的输入进行加密,请告诉我具体步骤和XAML。例如该在MainWindow.xaml中写什么,在MainWindow.xaml.cs中写什么
时间: 2024-12-11 21:42:16 浏览: 12
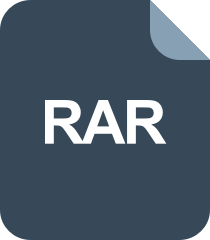
ChatGPT帮我用WPF制作一个简易贪吃蛇小游戏
在C# WPF中实现一个简单的加密算法,并将其集成到一个窗口中,通常需要经过以下几个步骤:
1. **添加必要的依赖项**:
在`MainWindow.xaml.cs`中添加System.Security.Cryptography命名空间,这个命名空间包含了加密相关的类。
```csharp
using System;
using System.Windows;
using System.Windows.Controls;
using System.Security.Cryptography;
```
2. **设计用户界面**:
在`MainWindow.xaml`中,你需要创建一个文本框用于接收用户输入和一个按钮用于触发加密过程。例如:
```xml
<Window x:Class="YourNamespace.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml">
<Grid>
<StackPanel>
<Label Content="请输入原始字符串:" />
<TextBox x:Name="InputTextBox" Width="200" Height="20"/>
<Button Content="加密" Click="Encrypt_Click"/>
</StackPanel>
</Grid>
</Window>
```
这里假设加密函数名为`Encrypt_Click`。
3. **编写加密函数**:
在`MainWindow.xaml.cs`中,为加密按钮的点击事件添加处理函数`Encrypt_Click()`。在这个函数中,获取输入值,然后应用加密算法。比如使用AES加密为例:
```csharp
private void Encrypt_Click(object sender, RoutedEventArgs e)
{
if (string.IsNullOrEmpty(InputTextBox.Text))
{
MessageBox.Show("请输入内容");
return;
}
string input = InputTextBox.Text;
byte[] key = Encoding.UTF8.GetBytes("your_secret_key_here"); // 替换为你自己的密钥
byte[] iv = new byte[16]; // AES使用16字节初始化向量
using (Aes aes = Aes.Create())
{
aes.Key = key;
aes.IV = iv;
ICryptoTransform encryptor = aes.CreateEncryptor(aes.Key, aes.IV);
using (MemoryStream msEncrypt = new MemoryStream())
{
using (CryptoStream csEncrypt = new CryptoStream(msEncrypt, encryptor, CryptoStreamMode.Write))
{
using (StreamWriter swEncrypt = new StreamWriter(csEncrypt))
{
swEncrypt.Write(input);
}
byte[] encryptedBytes = msEncrypt.ToArray();
InputTextBox.Clear(); // 清空输入框,防止显示明文
// 将加密后的二进制数据存储或展示在新的控件中,比如一个`TextBox`或`ByteArrayToHexBox`转换成十六进制显示
}
}
}
}
```
请注意替换上述示例中的密钥和IV,以及适当地处理结果。
阅读全文
相关推荐
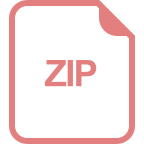
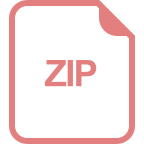
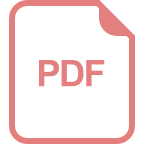
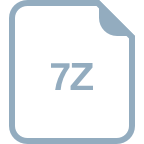
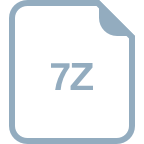
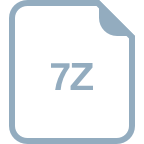