如何用图论的代码方式实现
时间: 2024-09-12 21:11:26 浏览: 29
在图论中,通常我们会使用邻接矩阵、邻接列表或者其他数据结构来表示图,并通过特定的数据结构和算法来操作它。以下是用Python的`networkx`库(一个流行用于图论的库)实现基本操作的一些例子:
1. **创建无向图**:
```python
import networkx as nx
# 创建一个无向图
G = nx.Graph()
G.add_edge(1, 2)
G.add_edge(2, 3)
```
2. **遍历邻居**:
```python
for node in G.nodes:
print(f"节点 {node} 的邻居是: {G[node]}")
```
3. **查找最短路径**:
```python
shortest_path = nx.shortest_path(G, source=1, target=4)
print(f"从1到4的最短路径是: {shortest_path}")
```
4. **检测连通分量**:
```python
components = list(nx.connected_components(G))
for component in components:
print(f"连通分量: {component}")
```
5. **求解最短路径树**:
```python
spanning_tree = nx.minimum_spanning_tree(G)
```
请注意,实际应用中,你可能还需要导入其他模块(如`matplotlib`用于可视化),并根据具体需求调整代码。在处理大型图或者复杂问题时,性能优化(例如使用适当的数据结构,或者采用并行化算法)也至关重要。
阅读全文
相关推荐
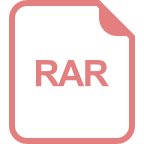
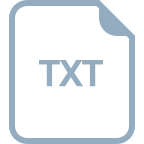
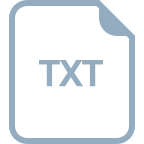
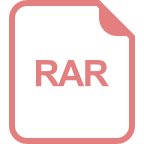
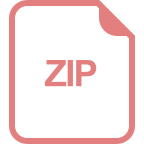
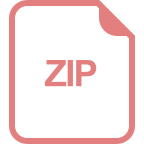
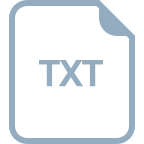
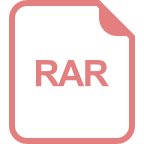
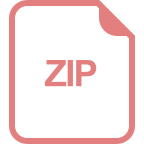
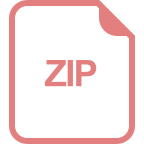
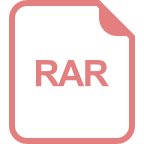
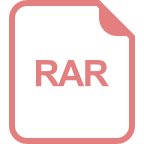
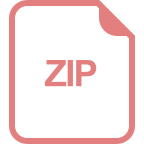
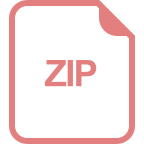
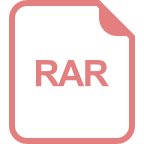
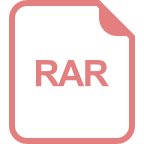
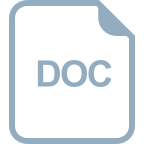
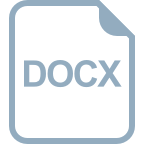