python 正则表达式怎么用
时间: 2024-01-25 09:12:10 浏览: 68
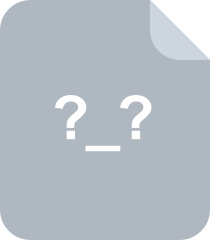
python中使用正则表达式
Python中使用正则表达式可以通过re库来实现。下面是一些常用的方法:
1. match()方法:从字符串的开头开始匹配,如果匹配成功,则返回一个匹配对象;如果匹配失败,则返回None。
```python
import re
pattern = r"hello"
string = "hello world"
result = re.match(pattern, string)
if result:
print("Match found!")
else:
print("Match not found!")
```
2. search()方法:在字符串中搜索匹配的内容,如果匹配成功,则返回一个匹配对象;如果匹配失败,则返回None。
```python
import re
pattern = r"world"
string = "hello world"
result = re.search(pattern, string)
if result:
print("Match found!")
else:
print("Match not found!")
```
3. findall()方法:在字符串中查找所有匹配的内容,并以列表的形式返回。
```python
import re
pattern = r"\d+"
string = "I have 123 apples and 456 oranges"
result = re.findall(pattern, string)
print(result) # 输出:['123', '456']
```
4. finditer()方法:在字符串中查找所有匹配的内容,并以迭代器的形式返回。
```python
import re
pattern = r"\d+"
string = "I have 123 apples and 456 oranges"
result = re.finditer(pattern, string)
for match in result:
print(match.group()) # 输出:123 456
```
5. sub()方法:替换字符串中匹配的内容。
```python
import re
pattern = r"apple"
string = "I have an apple"
result = re.sub(pattern, "orange", string)
print(result) # 输出:I have an orange
```
阅读全文
相关推荐
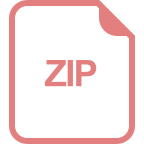
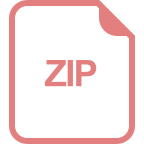











