5. 编程要求: - 输入年月,输出对应月份的日历。 - 思路:先解决“某年某月1日是星期几”,再根据月历规律递推该月每一天的分布。
时间: 2024-12-18 15:17:30 浏览: 8
要编写一个程序,根据给定的年月输出对应的日历,你可以按照以下思路进行:
1. **获取当前日期信息**:
首先,你需要获取当前的年份、月份和日期(如果你没有特定的输入),可以使用`time.h`库中的`time()`和`localtime()`函数。
```c
#include <stdio.h>
#include <time.h>
struct tm today = {};
time_t now = time(NULL);
localtime(&now, &today);
int year = today.tm_year + 1900; // Adjust for the Gregorian calendar
int month = today.tm_mon + 1;
```
2. **计算指定日期的星期**:
使用`zoneday()`函数从`<time.h>`库来计算某个日期是星期几。这里需要包含`<date.h>`头文件(可能需要编译器支持)。
```c
#include <date.h>
// Assuming `year`, `month` and `day` are valid
int day_of_week(int year, int month, int day) {
return zoneday(year, month, day, "UTC"); // Replace with your time zone if needed
}
```
3. **确定月份天数**:
根据月份和年份,确定本月有多少天。你可以创建一个数组或动态生成来存储每个月的天数,或者使用标准库中的`<ctime.h>`中的`mktime()`和`difftime()`来判断。
```c
#include <ctime.h>
const int days_in_month[] = {31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31}; // For non-leap years
int num_days_in_month(int year, int month) {
if (is_leap_year(year)) {
// Modify days_in_month for leap years
} else {
return days_in_month[month - 1];
}
}
bool is_leap_year(int year) {
return (year % 4 == 0 && year % 100 != 0) || (year % 400 == 0);
}
```
4. **生成并打印日历**:
创建一个二维数组(如7x5大小,表示一周7天,一个月最多有5个完整的行)来填充月份的日历,然后循环遍历每一天,用之前计算得到的星期几填充相应的格子。
```c
#define ROWS 7
#define COLS 5
void print_calendar(int year, int month) {
int days[] = {1};
int i, j;
for (i = 0; i < ROWS; ++i) {
for (j = 0; j < COLS && days[0] <= num_days_in_month(year, month); ++j, ++days[0]) {
printf("%2d ", days[0]);
}
if (j < COLS) {
// If not all days in a row fit, start a new week
printf("\n");
} else {
printf("\n");
}
// Move to next line
if (i % 6 == 5) {
printf("--\n");
}
}
}
int main() {
print_calendar(year, month);
return 0;
}
```
阅读全文
相关推荐
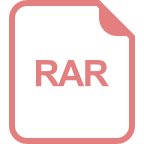
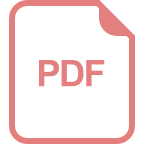
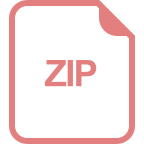
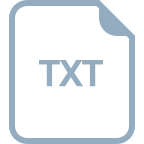
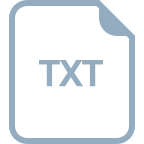
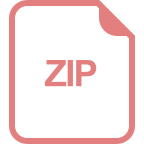
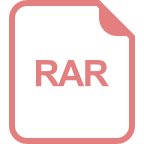
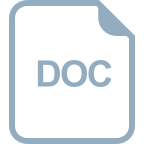
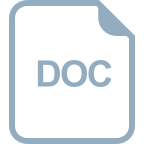
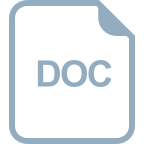
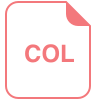
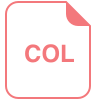
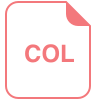
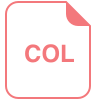
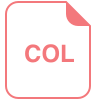
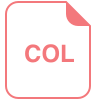
