java 多线程:编写一个程序,模拟3个买票窗口,共卖十张票,每张票卖一次
时间: 2024-10-12 21:12:25 浏览: 24
在Java中创建一个多线程模拟3个售票窗口的情况,我们可以使用`Thread`类、`Runnable`接口或者`ExecutorService`来实现并发。这里我将给出一个简单的示例,展示如何使用`Runnable`接口:
```java
import java.util.concurrent.atomic.AtomicInteger;
public class TicketWindow implements Runnable {
private static AtomicInteger ticketCount = new AtomicInteger(10); // 票的数量
private int windowId; // 窗口ID
public TicketWindow(int id) {
this.windowId = id;
}
@Override
public void run() {
while (ticketCount.get() > 0) { // 当还有票的时候
System.out.println("窗口 " + windowId + " 开始售票,当前剩余票数:" + ticketCount.get());
// 模拟购票过程,这里简单地减少票数
if ((ThreadLocalRandom.current().nextInt(1, 4) == 1)) { // 有1/4的概率有人购买
ticketCount.decrementAndGet(); // 减少一张票
System.out.println("窗口 " + windowId + " 卖出了一张票,剩余 " + ticketCount.get() + " 张");
} else {
System.out.println("窗口 " + windowId + " 没有人购买,等待下一位");
}
try {
Thread.sleep(500); // 模拟售票员休息时间
} catch (InterruptedException e) {
e.printStackTrace();
}
}
System.out.println("窗口 " + windowId + " 所有票已售完");
}
public static void main(String[] args) {
Thread thread1 = new Thread(new TicketWindow(1), "窗口1");
Thread thread2 = new Thread(new TicketWindow(2), "窗口2");
Thread thread3 = new Thread(new TicketWindow(3), "窗口3");
thread1.start();
thread2.start();
thread3.start();
// 等待所有窗口结束
try {
thread1.join();
thread2.join();
thread3.join();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
阅读全文
相关推荐
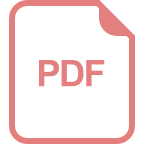
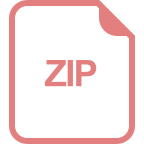
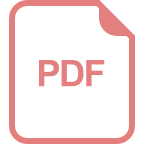















