用c语言在单链表上实现元素的有序插入
时间: 2024-09-25 18:14:57 浏览: 21
在C语言中,要在单链表上实现元素的有序插入,通常需要维护链表的结构,并确保新插入的节点保持链表原有的升序排列。这里是一个基本的步骤:
1. 首先,你需要定义一个结构体表示链表节点,包括数据域(存储元素值)和指针域(指向下一个节点)。
```c
typedef struct ListNode {
int data;
struct ListNode* next;
} ListNode;
```
2. 创建链表头指针,并初始化为空。
```c
ListNode* head = NULL;
```
3. 定义一个辅助函数来比较新节点的数据与现有链表中的节点,找到合适的位置插入。
```c
int compareNodes(ListNode* node1, ListNode* node2) {
return node1->data < node2->data ? -1 : (node1->data > node2->data ? 1 : 0);
}
```
4. 实现插入函数,遍历链表,找到适当位置插入新节点。
```c
void insertSortedNode(int value, ListNode** head) {
ListNode* newNode = (ListNode*)malloc(sizeof(ListNode));
newNode->data = value;
newNode->next = *head;
if (*head != NULL && compareNodes(newNode, *head) > 0) {
// 新节点大于头节点,直接插入到头节点前
newNode->next = *head->next;
*head = newNode;
} else {
ListNode* current = *head;
while (current->next != NULL && compareNodes(current->next, newNode) >= 0) {
current = current->next;
}
// 找到正确位置并插入
newNode->next = current->next;
current->next = newNode;
}
}
```
5. 使用这个`insertSortedNode`函数添加新的元素,例如:
```c
// 示例:将数字5、2和9依次插入到已经排序好的链表[1 -> 3 -> 4 -> 6]中
insertSortedNode(5, &head); // [1 -> 2 -> 3 -> 4 -> 5 -> 6]
insertSortedNode(2, &head); // [1 -> 2 -> 3 -> 4 -> 5 -> 6]
insertSortedNode(9, &head); // [1 -> 2 -> 3 -> 4 -> 5 -> 6 -> 9]
```
相关推荐
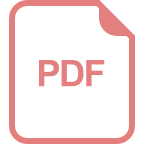
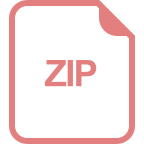
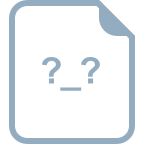
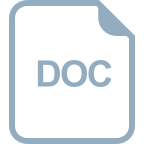
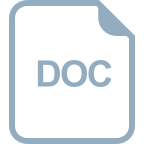

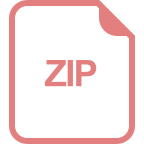
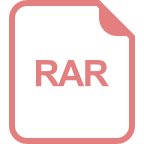
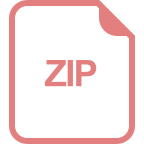
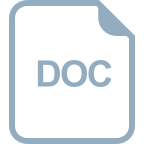
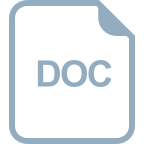
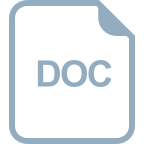
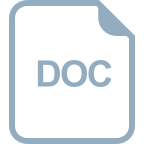
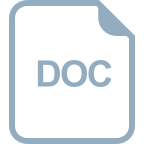
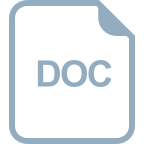


