yolov5加pyqt5
时间: 2024-12-30 16:22:29 浏览: 7
### 实现YOLOv5与PyQt5结合的目标检测GUI应用程序
#### 创建项目结构
为了使项目有条理,建议创建如下目录结构:
```
project/
│── yolov5/ # YOLOv5源码所在位置
│── gui/ # PyQt5 GUI代码所在的文件夹
│ ├── main.py # 主入口文件
│ └── ui_main_window.ui # Qt Designer设计的UI文件
└── requirements.txt # 依赖项列表
```
#### 安装依赖项
确保安装所需的Python库。`requirements.txt` 文件应包含必要的依赖关系,如 `torch`, `opencv-python-headless`, `pyqt5` 和其他YOLOv5所需组件。
```bash
pip install -r requirements.txt
```
#### 设计用户界面
使用Qt Designer来绘制用户界面,并保存为 `.ui` 文件。此界面应当至少具备以下元素:
- 打开摄像头按钮
- 显示视频帧的区域(QLabel)
- 开始/停止检测按钮
- 展示检测结果的文字框或标签
#### 编写核心逻辑
在 `main.py` 中编写主要业务逻辑,连接YOLO模型加载、图像处理以及事件响应等功能模块。
```python
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow
from PyQt5.uic import loadUi
import cv2
import torch
from pathlib import Path
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
# 加载UI定义
self.ui = loadUi('gui/ui_main_window.ui', self)
# 初始化YOLOv5模型
weights_path = 'yolov5s.pt'
model_dir = str(Path(__file__).parent / "yolov5")
self.model = torch.hub.load(model_dir, 'custom', path=weights_path, source='local')
# 绑定按钮点击事件处理器
self.open_camera_button.clicked.connect(self.start_video_capture)
self.detect_objects_button.clicked.connect(self.perform_detection)
def start_video_capture(self):
"""初始化并启动摄像头捕获"""
pass
def perform_detection(self):
"""执行目标检测并将结果显示出来"""
frame = ... # 获取当前帧
results = self.model(frame) # 进行预测
result_frame = results.render()[0] # 渲染带标注的结果图
height, width, channel = result_frame.shape
bytesPerLine = 3 * width
qImg = QImage(result_frame.data, width, height, bytesPerLine, QImage.Format_RGB888).rgbSwapped()
self.image_label.setPixmap(QPixmap.fromImage(qImg)) # 更新显示图片
if __name__ == '__main__':
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec_())
```
上述代码展示了如何设置基本的应用窗口和关联YOLOv5模型进行推理的过程[^1][^2]。
#### 测试与优化
完成初步编码后,运行程序测试各个部分的功能是否正常工作。根据实际需求调整参数配置,比如改变使用的预训练权重文件路径、修改界面样式等。
阅读全文
相关推荐


















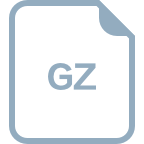