使用 Django Element vue.js axios 文件和字符串一起上传,并且使用中间件
时间: 2024-04-15 20:29:21 浏览: 216
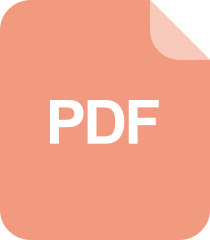
vue项目中使用axios上传图片等文件操作
您可以使用Django、Element UI、Vue.js和Axios来实现文件和字符串一起上传的功能,并使用中间件来处理请求。下面是一个简单的示例代码:
1. 首先,安装所需的库:
```
pip install django
npm install element-ui
npm install axios
```
2. 创建Django项目并设置好数据库。
3. 在Django项目的settings.py文件中,添加以下配置:
```python
INSTALLED_APPS = [
...
'corsheaders',
]
MIDDLEWARE = [
...
'corsheaders.middleware.CorsMiddleware',
]
CORS_ORIGIN_ALLOW_ALL = True
```
4. 创建Django视图(view)和路由(url)。
```python
# views.py
from django.http import JsonResponse
def upload_file(request):
file = request.FILES.get('file')
text = request.POST.get('text')
# 处理文件和字符串上传逻辑
return JsonResponse({'message': '上传成功'})
# urls.py
from django.urls import path
from . import views
urlpatterns = [
path('upload/', views.upload_file, name='upload_file'),
]
```
5. 在Vue组件中,使用Element UI和Axios发送POST请求来上传文件和字符串。
```vue
<template>
<div>
<el-upload
action="/upload/"
:on-success="handleSuccess"
:before-upload="beforeUpload"
:auto-upload="false"
:headers="headers"
>
<el-button slot="trigger" size="small" type="primary">选择文件</el-button>
</el-upload>
<el-input v-model="text"></el-input>
<el-button @click="upload">上传</el-button>
</div>
</template>
<script>
import axios from 'axios';
export default {
data() {
return {
file: null,
text: '',
headers: {
'Content-Type': 'multipart/form-data',
},
};
},
methods: {
beforeUpload(file) {
this.file = file;
return false; // 阻止自动上传
},
upload() {
const formData = new FormData();
formData.append('file', this.file);
formData.append('text', this.text);
axios.post('/upload/', formData, {
headers: this.headers,
})
.then(response => {
console.log(response.data.message);
})
.catch(error => {
console.error(error);
});
},
handleSuccess(response) {
console.log(response);
},
},
};
</script>
```
6. 在Vue应用中引入Element UI和Axios。
```js
// main.js
import Vue from 'vue';
import ElementUI from 'element-ui';
import 'element-ui/lib/theme-chalk/index.css';
Vue.use(ElementUI);
```
这样,您就可以通过Element UI的上传组件将文件和字符串一起上传到Django服务器了。在Django视图中的upload_file函数中,您可以根据需要对文件和字符串进行处理。
阅读全文
相关推荐
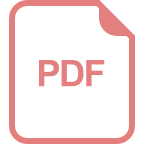
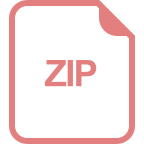
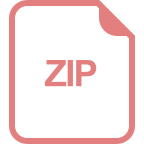
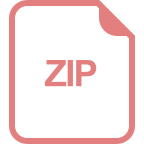
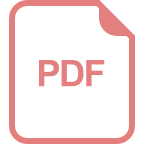
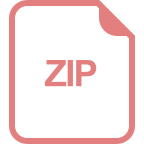
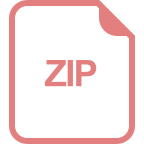
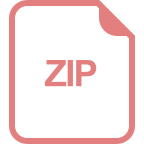
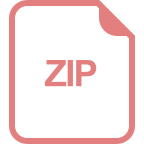
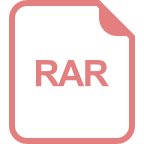
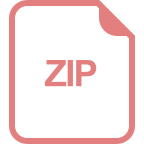
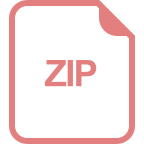
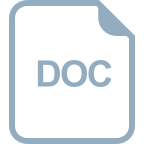
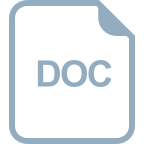
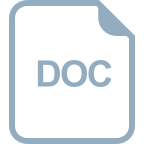
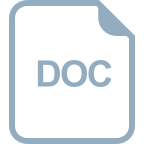
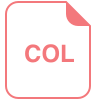