Android MVVM app如何重启
时间: 2024-09-13 20:04:37 浏览: 48
在Android中,如果你需要重启一个使用MVVM架构的应用程序,你通常需要终止当前的Activity堆栈,并重新启动应用程序的主入口点。以下是一种方法:
1. 使用Intent重新启动应用程序的主Activity:
```java
Intent intent = getPackageManager().getLaunchIntentForPackage(getPackageName());
intent.addFlags(Intent.FLAG_ACTIVITY_CLEAR_TOP); // 清除当前任务栈上的所有Activity
intent.addFlags(Intent.FLAG_ACTIVITY_NEW_TASK); // 创建新的任务栈
startActivity(intent);
```
2. 退出当前应用程序:
```java
finishAffinity(); // 结束当前Activity及其所有父Activity
android.os.Process.killProcess(android.os.Process.myPid()); // 杀死当前应用程序进程
```
请注意,强制关闭应用程序并不是一个好的用户体验,通常应避免在正常情况下使用这种做法。在大多数情况下,Android系统会管理应用程序的生命周期,当系统需要时会自动重启应用程序。
相关问题
android mvvm
MVVM (Model-View-ViewModel) is a design pattern that is widely used in Android app development. It separates the UI (View) from the business logic (Model) and adds an intermediate layer (ViewModel) that handles the communication between the two.
In an MVVM architecture, the Model represents the data and the business logic of the app. The ViewModel acts as a mediator between the Model and the View. It receives data from the Model and exposes it to the View in a way that can be easily consumed. The View is responsible for displaying the data to the user and handling user input.
The benefits of using MVVM in Android app development include:
1. Separation of concerns: MVVM separates the UI logic from the business logic, making the code easier to maintain and test.
2. Testability: With MVVM, the ViewModel can be easily tested without needing access to the UI.
3. Scalability: MVVM allows for easier scaling of the app as it grows in complexity and size.
4. Flexibility: With MVVM, you can easily switch out the View or Model without affecting the other components.
Overall, MVVM is a powerful pattern that can help developers create more robust and maintainable Android apps.
android mvvm示例
好的,以下是一个简单的 Android MVVM 示例:
1. 创建一个 ViewModel 类
```kotlin
import androidx.lifecycle.MutableLiveData
import androidx.lifecycle.ViewModel
class MainViewModel : ViewModel() {
val message = MutableLiveData<String>()
init {
message.value = "Hello World!"
}
}
```
这个 ViewModel 类通过 LiveData 提供了一个可观察的字符串数据。在初始化过程中,将这个字符串设置为 "Hello World!"。
2. 在布局文件中添加 DataBinding
```xml
<layout xmlns:android="http://schemas.android.com/apk/res/android">
<data>
<variable
name="viewModel"
type="com.example.MainViewModel" />
</data>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="@{viewModel.message}" />
</LinearLayout>
</layout>
```
这个布局文件使用了 DataBinding,它包含一个 TextView,它的文本属性绑定到了 ViewModel 中的 message 属性。
3. 在 Activity 中设置 ViewModel 和 DataBinding
```kotlin
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import androidx.databinding.DataBindingUtil
import androidx.lifecycle.ViewModelProvider
import com.example.databinding.ActivityMainBinding
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
val binding: ActivityMainBinding = DataBindingUtil.setContentView(this, R.layout.activity_main)
val viewModel = ViewModelProvider(this).get(MainViewModel::class.java)
binding.viewModel = viewModel
}
}
```
这个 Activity 使用了 DataBinding,它通过 ViewModelProvider 获取 ViewModel 实例,并将 ViewModel 实例设置到 DataBinding 中。这样,ViewModel 中的数据就可以与视图进行绑定了。
这个示例中,ViewModel 中的 message 属性绑定到了 TextView 的文本属性,因此当 ViewModel 中的 message 属性发生改变时,TextView 的文本也会相应改变。这就是 MVVM 架构的核心思想:将视图与数据通过 ViewModel 进行绑定,实现视图和数据的解耦合,使得代码更加清晰和易于维护。
阅读全文
相关推荐
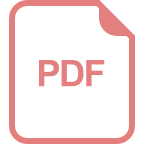
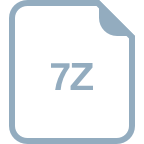
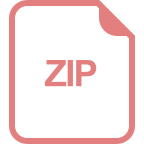
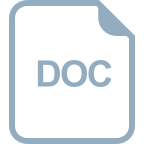
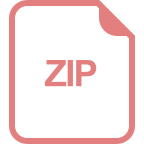
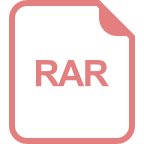
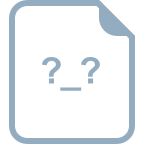
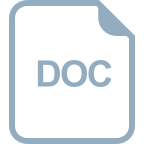
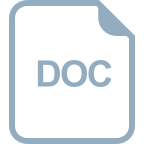

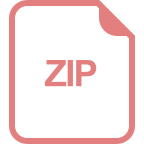
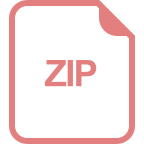
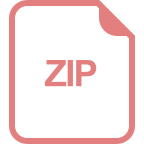
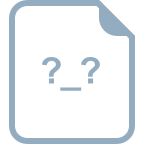
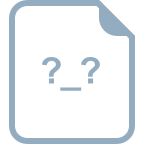
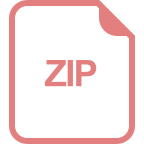
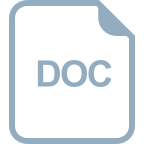
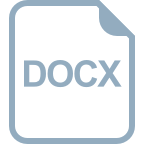