arduino控制gsm模块发送短信报警 的代码
时间: 2024-09-28 19:06:55 浏览: 38
Arduino 控制 GSM 模块发送短信报警通常涉及硬件连接、初始化通信以及编写特定的代码片段来实现这一功能。以下是一个简单的示例,假设你正在使用 Arduino Uno 和一个支持 AT 命令的 SMS/GSM 模块如 SIM800L 或者其同类产品。
1. **硬件准备**:
- 连接 GSM 模块的 TX/RX 端口到 Arduino 的 RX/TX 引脚(例如 RX-0/GND 和 TX-1/GND)。
- 如果需要电源,连接电池或外部电源适配器到模块的 VCC 和 GND 接口。
2. **软件库安装**:
- 首先,你需要下载并安装 Arduino IDE,然后从 Library Manager 中安装 "SoftwareSerial" 库,以便在 Uno 类型的板子上模拟串行通信。
3. **初始化代码**:
```cpp
#include <SoftwareSerial.h>
SoftwareSerial gsm(2, 3); // RX (pin 2), TX (pin 3) for GSM module
void setup() {
Serial.begin(9600); // Initialize serial communication for debugging
gsm.begin(9600); // Start the SoftwareSerial connection to GSM module
delay(2000); // Give GSM time to initialize
// Send initialization commands if needed (e.g., AT command to set up SMS mode)
}
void loop() {
String message = "ALARM DETECTED"; // The text you want to send
if (isAlarmTriggered()) { // Replace this with your actual alarm detection logic
sendSMS(message);
}
delay(5000); // Check for alarms every few seconds
}
// Function to send an SMS
void sendSMS(String text) {
gsm.println("AT+CMGF=1"); // Set SMS format to TEXT (0 for PDU)
delay(1000);
gsm.println("AT+CMGS=\"tel_number\"");
delay(1000);
gsm.print(text);
gsm.println((char)26); // ASCII code for Ctrl+Z (end of transmission)
delay(1000); // Wait before reading response
}
```
4. **注意**:
- 在实际应用中,你需要替换 `sendSMS` 函数中的 `"tel_number"` 为你想要发送短信的实际电话号码。
- GSM 模块可能会返回一些响应,比如错误代码,确保处理这些情况。
- `isAlarmTriggered()` 是一个假设的函数,你需要实现你的报警触发条件。
阅读全文
相关推荐
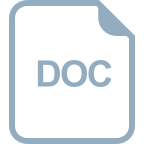
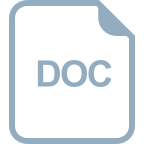
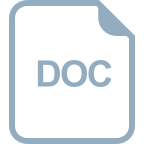
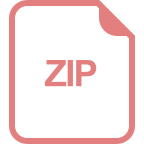
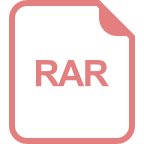
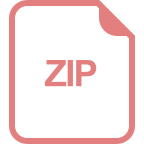
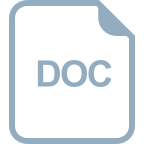
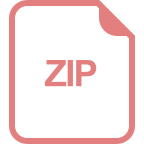
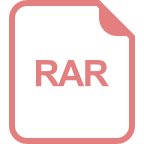
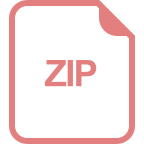
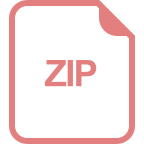
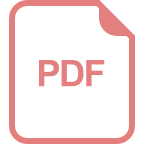
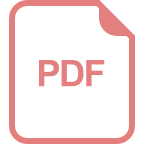
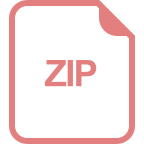
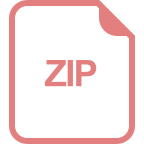
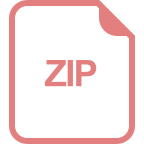
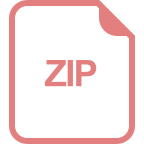
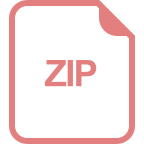
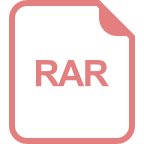