实现单链表的定义和遍历和求长度和按序号查找和插入和删除的代码
时间: 2024-10-11 11:07:42 浏览: 77
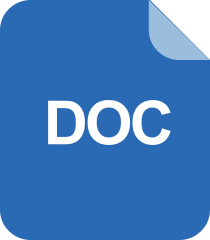
单链表的创建和插入

在许多编程语言中,特别是像C++或Python这样的面向对象语言,我们可以创建一个单链表的数据结构来存储一系列元素,并提供相应的操作。下面是一个简单的C++单链表实现及其常见操作:
```cpp
// 定义链表节点
class ListNode {
public:
int val; // 节点值
ListNode* next; // 指向下一个节点的指针
ListNode(int x) : val(x), next(nullptr) {} // 构造函数
};
// 单链表的基本操作
class LinkedList {
private:
ListNode* head;
public:
// 初始化空链表
LinkedList() : head(nullptr) {}
// 遍历链表(仅用于演示,实际应用中通常需要自定义迭代器)
void traverse() {
ListNode* current = head;
while (current != nullptr) {
cout << current->val << " ";
current = current->next;
}
cout << endl;
}
// 计算链表长度
int length() {
int count = 0;
ListNode* temp = head;
while (temp != nullptr) {
count++;
temp = temp->next;
}
return count;
}
// 顺序查找(若存在则返回,不存在返回nullptr)
ListNode* search(int target) {
ListNode* current = head;
while (current != nullptr && current->val != target) {
current = current->next;
}
return current;
}
// 在特定位置插入节点
void insert(int index, int value) {
if (index <= 0 || index > length()) {
cout << "Invalid index." << endl;
return;
}
ListNode* newNode = new ListNode(value);
if (index == 1) { // 如果在头部插入
newNode->next = head;
head = newNode;
} else {
ListNode* prevNode = nullptr;
for (int i = 0; i < index - 1 && head != nullptr; ++i) {
prevNode = head;
head = head->next;
}
if (prevNode) {
newNode->next = head;
prevNode->next = newNode;
}
}
}
// 删除指定位置的节点(如果存在)
bool remove(int index) {
if (index <= 0 || index > length()) {
cout << "Invalid index." << endl;
return false;
}
if (index == 1) { // 如果删除头节点
ListNode* temp = head;
head = head->next;
delete temp;
return true;
} else {
ListNode* current = head;
for (int i = 1; i < index && current != nullptr; ++i) {
current = current->next;
}
if (current) {
ListNode* nextNode = current->next;
delete current;
current->next = nextNode;
return true;
}
}
return false;
}
};
阅读全文
相关推荐
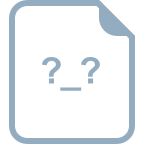
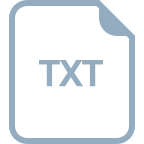
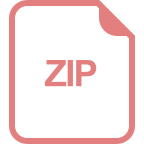
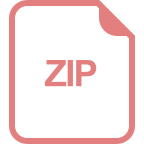
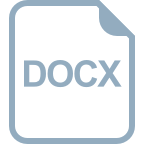
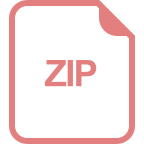
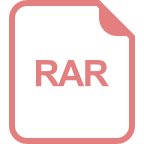
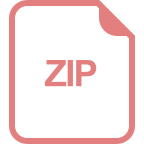
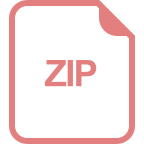