list indices must be integers or slices, not tuple; perhaps you missed a comma?
时间: 2024-06-01 11:05:53 浏览: 187
这个错误通常出现在你想要使用一个tuple作为list的索引时。在Python中,list的索引只能是整数或者切片,不能是tuple。
例如,以下代码会产生这个错误:
```
my_list = [1, 2, 3, 4, 5]
index = (1, 2)
print(my_list[index])
```
要解决这个错误,你需要将tuple转换成整数或者切片来作为list的索引。比如,如果你想要使用(1,2)作为索引,你可以这样做:
```
my_list = [1, 2, 3, 4, 5]
index = (1, 2)
print(my_list[index], my_list[index])
```
相关问题
SyntaxWarning: list indices must be integers or slices, not tuple; perhaps you missed a comma?
This warning occurs when trying to use a tuple as an index for a list. In Python, list indices must be integers or slices, not tuples.
For example, if we have a list of lists and we want to access an element in a specific sublist using a tuple as an index, we will get this warning:
```
list_of_lists = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
# Trying to access an element in a specific sublist using a tuple as an index
sublist_index = (1, 2)
element = list_of_lists[sublist_index] # Raises a SyntaxWarning
```
To fix this warning, we need to use a comma to separate the tuple elements and convert it to an integer index:
```
list_of_lists = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
# Accessing an element in a specific sublist using an integer index
sublist_index = 1
element = list_of_lists[sublist_index][2] # Returns 6
```
SyntaxWarning: list indices must be integers or slices, not tuple; perhaps you missed a comma?怎么解决
这个警告通常是由于在使用列表时,索引使用了元组而不是整数或切片。要解决这个问题,你需要检查代码中的索引操作,并确保使用的是整数或切片。
以下是一些可能导致这个警告的常见情况和解决方法:
1. 检查索引操作是否使用了元组而不是整数或切片。例如,`my_list[1, 2]`应该改为`my_list`或`my_list[1:2]`。
2. 如果你使用了多个索引操作,确保每个索引操作都是整数或切片。例如,`my_list[2, 3]`应该改为`my_list`或`my_list[1:2]`。
3. 如果你使用了变量作为索引,确保这些变量的值是整数或切片。例如,`index = (1, 2)`应该改为`index = 1, 2`。
如果以上方法都没有解决问题,那么可能是代码其他部分导致了这个警告。你可以提供更多的代码细节,以便我能够更好地帮助你解决问题。
相关推荐
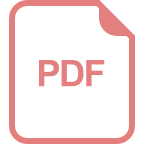
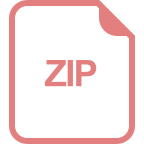












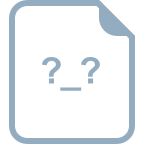
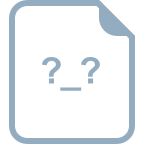
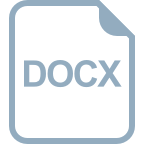