c++蚁群算法旅行商
时间: 2024-06-04 15:04:43 浏览: 14
蚁群算法是一种基于自然界中蚂蚁觅食行为的一种启发式算法,应用广泛,其中最著名的应用之一就是求解旅行商问题。旅行商问题是指,给定一些城市和每两个城市之间的距离,找出访问每个城市一次且仅一次的最短路径。而使用蚁群算法来求解旅行商问题时,可以通过模拟蚂蚁在城市间的移动来寻找最优解。具体来说,可以将每只蚂蚁看作一个解决方案的生成器,在搜索空间中寻找最优解。
C++是一种流行的编程语言,因其高效性和广泛应用而受到广泛关注。在C++中,实现蚁群算法求解旅行商问题可以采用多种方式,例如利用结构体存储城市和距离信息、使用类实现蚂蚁等。
相关问题
qt蚁群算法旅行商问题c++
QT是一款跨平台的GUI应用程序开发框架,而蚁群算法是一种优化算法。旅行商问题是指一个旅行商要前往n个城市,必须恰好访问每个城市一次,并且最终回到出发城市。问题的目标是确定一条路径,使得路径的总长度最小。
在QT中实现蚁群算法解决旅行商问题的过程,可以分为以下几个步骤:
1.初始化蚁群:随机生成初始解,即每只蚂蚁随机选择一个起始城市。
2.计算信息素:每只蚂蚁根据当前城市和信息素浓度选择下一个城市,选择的概率与信息素浓度有关。
3.更新信息素:每只蚂蚁走完一条路径后,更新路径上经过的边上的信息素浓度。
4.判断终止条件:当满足一定条件时,停止迭代。
5.输出结果:输出最优解。
以下是C++代码示例:
```
#include <iostream>
#include <cstring>
#include <cmath>
#include <cstdlib>
#include <ctime>
using namespace std;
const int city_num = 48; //城市数量
const int ant_num = 100; //蚂蚁数量
double alpha = 1.0; //信息素重要程度因子
double beta = 5.0; //启发函数重要程度因子
double rho = 0.5; //信息素挥发因子
double Q = 100.0; //常系数
double distance[city_num][city_num]; //两两城市间距离
double pheromone[city_num][city_num]; //两两城市间信息素浓度
int best_ant[city_num + 1]; //记录最优路径
double best_length = 1e9; //记录最优路径长度
double ant_distance[ant_num]; //记录每只蚂蚁的路径长度
void init() { //初始化函数
srand(time(NULL));
for (int i = 0; i < city_num; i++)
for (int j = 0; j < city_num; j++) {
distance[i][j] = rand() % 100 + 1;
pheromone[i][j] = 1.0;
}
}
double heuristic(int from, int to) { //启发函数,计算两个城市间的启发值
return 1.0 / distance[from][to];
}
int choose_next_city(int ant, bool *visited) { //选择下一个城市
double p[city_num];
memset(p, 0, sizeof(p));
int current_city = best_ant[ant];
double sum = 0.0;
for (int i = 0; i < city_num; i++) {
if (!visited[i]) {
p[i] = pow(pheromone[current_city][i], alpha) * pow(heuristic(current_city, i), beta);
sum += p[i];
}
}
double r = (double) rand() / RAND_MAX;
double tmp = 0.0;
for (int i = 0; i < city_num; i++) {
if (!visited[i]) {
tmp += p[i] / sum;
if (r <= tmp) {
return i;
}
}
}
return -1;
}
void update_pheromone() { //更新信息素浓度
for (int i = 0; i < city_num; i++)
for (int j = 0; j < city_num; j++) {
pheromone[i][j] *= (1 - rho);
for (int k = 0; k < ant_num; k++)
pheromone[i][j] += Q / ant_distance[k] * (best_ant[k] == i && best_ant[k + 1] == j);
}
}
void ant_colony() { //蚁群算法主函数
for (int iter = 0; iter < 100; iter++) { //迭代次数
for (int ant = 0; ant < ant_num; ant++) { //每只蚂蚁
bool visited[city_num];
memset(visited, false, sizeof(visited));
best_ant[ant] = rand() % city_num;
visited[best_ant[ant]] = true;
for (int i = 1; i < city_num; i++) { //选择下一个城市
int next_city = choose_next_city(ant, visited);
visited[next_city] = true;
ant_distance[ant] += distance[best_ant[ant]][next_city];
best_ant[ant + 1] = next_city;
best_length = min(best_length, ant_distance[ant]);
}
ant_distance[ant] += distance[best_ant[ant]][best_ant[city_num - 1]];
}
update_pheromone(); //更新信息素浓度
}
}
int main() {
init();
ant_colony();
cout << "Best Length: " << best_length << endl;
cout << "Best Path: ";
for (int i = 0; i <= city_num; i++)
cout << best_ant[i] << " ";
cout << endl;
return 0;
}
```
用蚁群算法解决旅行商问题c++
蚁群算法是一种模拟蚂蚁觅食行为的启发式算法,可以用于解决旅行商问题(TSP)。下面是一个用C++实现蚁群算法解决旅行商问题的示例代码:
```cpp
#include <iostream>
#include <vector>
#include <cmath>
#include <cstdlib>
#include <ctime>
using namespace std;
const int MAX_CITIES = 10; // 城市数量
const int MAX_ANTS = 10; // 蚂蚁数量
const int MAX_ITERATIONS = 100; // 最大迭代次数
const double ALPHA = 1.0; // 信息素重要程度因子
const double BETA = 2.0; // 启发函数重要程度因子
const double RHO = 0.5; // 信息素蒸发系数
const double Q = 100.0; // 信息素增加强度系数
struct City {
double x, y;
};
double distance(const City& city1, const City& city2) {
double dx = city1.x - city2.x;
double dy = city1.y - city2.y;
return sqrt(dx * dx + dy * dy);
}
class Ant {
public:
Ant() {
tabu.resize(MAX_CITIES, false);
path.resize(MAX_CITIES);
}
void clear() {
for (int i = 0; i < MAX_CITIES; ++i) {
tabu[i] = false;
path[i] = 0;
}
}
void visitCity(int city) {
tabu[city] = true;
path[currentCity] = city;
currentCity = city;
tourLength += distance(cities[path[currentCity]], cities[path[currentCity - 1]]);
}
int getCurrentCity() const {
return currentCity;
}
double getTourLength() const {
return tourLength;
}
void setCurrentCity(int city) {
currentCity = city;
}
private:
vector<bool> tabu;
vector<int> path;
int currentCity = 0;
double tourLength = 0.0;
};
class ACO {
public:
ACO() {
cities.resize(MAX_CITIES);
ants.resize(MAX_ANTS);
pheromone.resize(MAX_CITIES, vector<double>(MAX_CITIES, 1.0));
// 初始化城市坐标
for (int i = 0; i < MAX_CITIES; ++i) {
cities[i].x = rand() % 100;
cities[i].y = rand() % 100;
}
// 初始化蚂蚁
for (int i = 0; i < MAX_ANTS; ++i) {
ants[i].clear();
ants[i].setCurrentCity(rand() % MAX_CITIES);
}
}
void updatePheromone() {
for (int i = 0; i < MAX_CITIES; ++i) {
for (int j = 0; j < MAX_CITIES; ++j) {
pheromone[i][j] *= (1.0 - RHO);
}
}
for (int i = 0; i < MAX_ANTS; ++i) {
for (int j = 0; j < MAX_CITIES; ++j) {
int city1 = ants[i].path[j];
int city2 = ants[i].path[(j + 1) % MAX_CITIES];
pheromone[city1][city2] += Q / ants[i].getTourLength();
pheromone[city2][city1] += Q / ants[i].getTourLength();
}
}
}
void antColonyOptimization() {
for (int iteration = 0; iteration < MAX_ITERATIONS; ++iteration) {
for (int i = 0; i < MAX_ANTS; ++i) {
while (ants[i].getCurrentCity() != -1) {
int nextCity = selectNextCity(ants[i]);
ants[i].visitCity(nextCity);
}
if (ants[i].getTourLength() < bestTourLength) {
bestTourLength = ants[i].getTourLength();
bestTour = ants[i].path;
}
ants[i].clear();
ants[i].setCurrentCity(rand() % MAX_CITIES);
}
updatePheromone();
}
}
int selectNextCity(const Ant& ant) {
int currentCity = ant.getCurrentCity();
double sum = 0.0;
for (int i = 0; i < MAX_CITIES; ++i) {
if (!ant.tabu[i]) {
sum += pow(pheromone[currentCity][i], ALPHA) * pow(1.0 / distance(cities[currentCity], cities[i]), BETA);
}
}
double r = (double)rand() / RAND_MAX;
double probability = 0.0;
for (int i = 0; i < MAX_CITIES; ++i) {
if (!ant.tabu[i]) {
probability += pow(pheromone[currentCity][i], ALPHA) * pow(1.0 / distance(cities[currentCity], cities[i]), BETA) / sum;
if (r <= probability) {
return i;
}
}
}
return -1;
}
void printBestTour() {
cout << "Best tour: ";
for (int i = 0; i < MAX_CITIES; ++i) {
cout << bestTour[i] << " ";
}
cout << endl;
cout << "Best tour length: " << bestTourLength << endl;
}
private:
vector<City> cities;
vector<Ant> ants;
vector<vector<double>> pheromone;
vector<int> bestTour;
double bestTourLength = numeric_limits<double>::max();
};
int main() {
srand(time(nullptr));
ACO aco;
aco.antColonyOptimization();
aco.printBestTour();
return 0;
}
```
这段代码实现了蚁群算法解决旅行商问题。它使用了随机生成的城市坐标作为输入,通过迭代更新信息素矩阵和蚂蚁的路径来寻找最优的旅行路径。最终输出最优路径和路径长度。
相关推荐
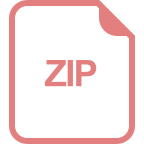
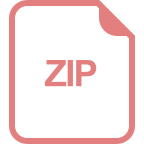
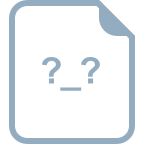










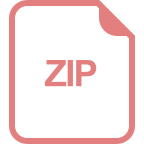
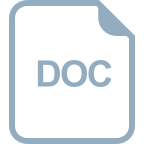