springmvc持久层代码
时间: 2024-07-01 12:00:59 浏览: 114
Spring MVC 中的持久层通常指的是数据访问层,使用 Spring JDBC、JPA 或 Hibernate 等技术。这里我会简单概述一下如何编写 Spring MVC 与 JPA(Java Persistence API)结合的持久层代码:
1. 添加依赖:在 Maven 或 Gradle 项目中添加 JPA 和 Hibernate 的依赖。
```xml
<!-- Maven -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<!-- Gradle (Kotlin) -->
implementation 'org.springframework.boot:spring-boot-starter-data-jpa'
```
2. 配置数据源:在 `application.properties` 或 `application.yml` 中配置数据库连接信息。
```properties
spring.datasource.url=jdbc:mysql://localhost:3306/your_database
spring.datasource.username=your_username
spring.datasource.password=your_password
spring.jpa.hibernate.ddl-auto=update
```
3. 定义 Entity 类(实体类):继承 `javax.persistence.Entity` 并添加属性和 ID。
```java
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
// getters and setters
}
```
4. 创建 Repository 接口:使用 Spring Data JPA 提供的仓库模式。
```java
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.stereotype.Repository;
@Repository
public interface UserRepository extends JpaRepository<User, Long> {
// CRUD operations
User findByEmail(String email);
}
```
5. Service 层:注入 Repository,并执行业务逻辑。
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class UserService {
private final UserRepository userRepository;
@Autowired
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
public User getUserByEmail(String email) {
return userRepository.findByEmail(email);
}
// Other methods...
}
```
6. 控制器层:调用 Service 方法并处理请求。
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class UserController {
private final UserService userService;
@Autowired
public UserController(UserService userService) {
this.userService = userService;
}
@GetMapping("/users/{email}")
public User getUser(@PathVariable("email") String email) {
return userService.getUserByEmail(email);
}
// Other endpoints...
}
```
阅读全文
相关推荐
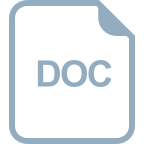
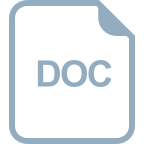
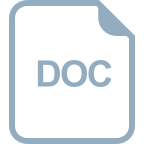
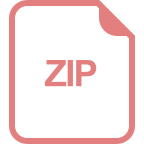
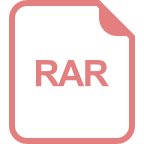
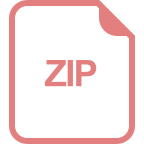
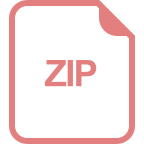
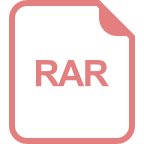
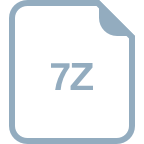
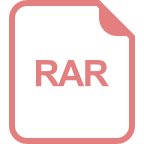
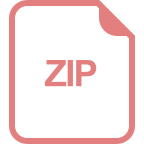
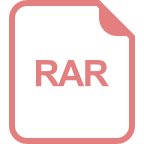
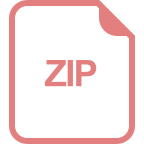
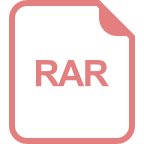
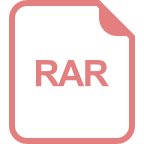
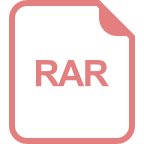
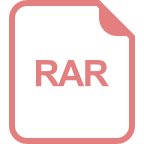
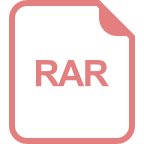
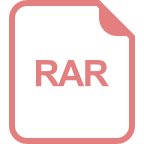