python画图 添加线条名称
时间: 2024-04-24 09:27:39 浏览: 124
要在Python画图中添加线条名称,可以使用matplotlib库中的label参数。在代码中使用plt.plot()函数时,可以通过label参数为每条线条添加名称。例如,可以按照以下方式修改代码来添加线条名称:
import matplotlib.pyplot as plt
import numpy as np
list1=[1,2,3,4,5,6,2,3,4,6,7,5]
list2=[2,4,4,5,8,9,2,1,3,4,5,2]
x=np.arange(0,len(list1))
plt.plot(x,list1,label='list1') # 添加label设置图例名称
plt.plot(x,list2,label='list2') # 添加label设置图例名称
plt.legend() # 显示图例
plt.show()
在上述代码中,通过在plt.plot()函数中使用label参数为每条线条添加名称。然后使用plt.legend()函数来显示图例。这样就可以在图中添加线条名称了。
相关问题
python 画图 五种蓝色的线条
好的,以下是使用Python绘制五种不同蓝色线条的代码:
```python
import matplotlib.pyplot as plt
import numpy as np
# 生成x和y的值
x = np.linspace(0, 10, 100)
y1 = np.sin(x)
y2 = np.cos(x)
y3 = np.tan(x)
y4 = np.exp(x)
y5 = np.log(x)
# 绘制图形
plt.plot(x, y1, color='blue', label='sin')
plt.plot(x, y2, color='skyblue', label='cos')
plt.plot(x, y3, color='cornflowerblue', label='tan')
plt.plot(x, y4, color='deepskyblue', label='exp')
plt.plot(x, y5, color='royalblue', label='log')
# 添加标题和标签
plt.title('Five Blue Lines')
plt.xlabel('x-axis')
plt.ylabel('y-axis')
plt.legend()
# 显示图形
plt.show()
```
以上代码中,使用了`color`参数来指定线条的颜色,具体的颜色值可以使用颜色名称或者RGB值来指定。在这里,我使用了五种不同的蓝色来绘制线条,它们分别是:
- `'blue'`
- `'skyblue'`
- `'cornflowerblue'`
- `'deepskyblue'`
- `'royalblue'`
这些颜色的深浅程度不同,可以让图形更加丰富,也可以根据自己的需要来调整。
阅读全文
相关推荐
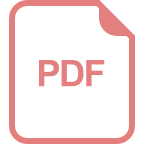
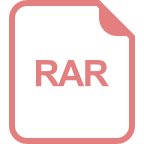
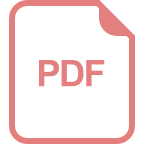
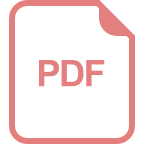
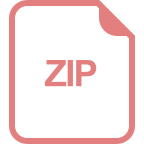
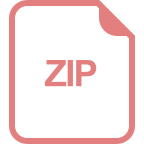
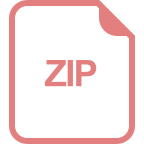
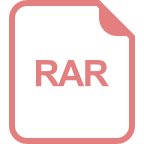
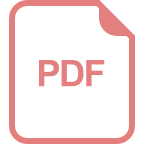
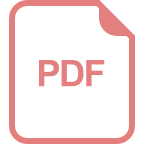
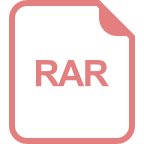
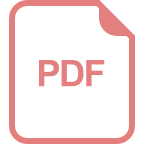
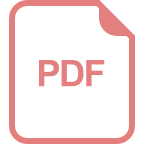
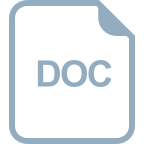
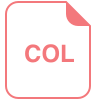
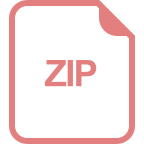