Linux 测试脚本执行
时间: 2024-09-05 07:04:17 浏览: 58
Linux测试脚本通常是指使用shell、bash或其他脚本语言编写的一系列命令,用于自动化执行系统、软件或应用程序的测试过程。这些脚本可以帮助开发者验证功能、性能、兼容性和安全性等方面。在Linux下,常见的测试脚本执行步骤包括:
1. **脚本编写**:使用文本编辑器创建一个.sh文件,内容包含一系列命令,如检查环境变量、运行程序、处理输出结果等。
2. **设置执行权限**:使用`chmod +x script_name.sh`确保脚本有执行权限。
3. **执行脚本**:通过`. /script_name.sh`或者直接运行`./script_name.sh`来运行测试脚本。
4. **日志记录**:通常会在脚本中加入日志输出,以便追踪测试进度和结果,可以使用`echo`、`>> log_file.log`等操作。
5. **条件控制**:使用if-else语句、循环结构(for、while)实现错误处理和逻辑判断。
6. **断言和验证**:利用工具如expect、assert等确认预期的行为是否发生。
7. **自动化框架集成**:例如JUnit、Selenium等可以与Linux脚本结合,提供更高级别的测试能力。
8. **持续集成/持续部署(CI/CD)**:将测试脚本纳入构建流程,确保代码提交后自动执行测试并反馈结果。
相关问题
linux线程栅栏测试脚本
以下是一个简单的 Linux 线程栅栏测试脚本示例,使用 pthread 库来创建和管理线程:
```bash
#!/bin/bash
# Set number of threads and iterations
NUM_THREADS=4
NUM_ITERATIONS=10
# Compile the test program
gcc -o thread_barrier_test thread_barrier_test.c -lpthread
# Run the test program
for ((i=0; i<$NUM_ITERATIONS; i++))
do
echo "Iteration: $i"
./thread_barrier_test $NUM_THREADS
done
# Clean up
rm thread_barrier_test
```
在此示例中,我们使用 gcc 编译了一个名为 thread_barrier_test 的可执行文件,该文件是使用 pthread 库编写的测试程序。我们将循环运行该程序 $NUM_ITERATIONS 次,每次输入不同的 $NUM_THREADS 线程数。在每个迭代中,我们都会向控制台输出一条消息以指示当前迭代编号,并启动测试程序。
测试程序首先使用 pthread 库创建 $NUM_THREADS 个线程,并在这些线程之间使用线程栅栏同步。然后,它会在控制台输出一条消息以指示线程已经开始运行,并在所有线程都完成其任务后输出另一条消息。
当运行测试程序时,您可以观察到线程栅栏同步的效果。如果测试程序正确实现了线程栅栏,那么所有线程都应该在完成其任务之前等待,然后同时完成并输出完成消息。
linux自旋锁测试脚本
以下是一个简单的 Linux 自旋锁测试脚本,它包含两个线程,一个线程获取锁并保持 5 秒钟,另一个线程在此期间尝试获取锁。脚本使用 shell 和 C 编写,需要安装 gcc 和 make。
```bash
#!/bin/bash
# Compile the C program
make spinlock
# Run the program with two threads
./spinlock 2 &
# Wait for the program to finish
wait
# Clean up
make clean
```
下面是 C 代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
pthread_spinlock_t lock;
void *thread_func(void *arg) {
int id = *(int *) arg;
int i;
// Try to acquire the lock
for (i = 0; i < 5; i++) {
printf("Thread %d trying to acquire lock\n", id);
pthread_spin_lock(&lock);
printf("Thread %d acquired lock\n", id);
sleep(1);
pthread_spin_unlock(&lock);
printf("Thread %d released lock\n", id);
sleep(1);
}
return NULL;
}
int main(int argc, char *argv[]) {
int num_threads = atoi(argv[1]);
int i;
// Initialize the spinlock
pthread_spin_init(&lock, 0);
// Create threads
pthread_t threads[num_threads];
int thread_ids[num_threads];
for (i = 0; i < num_threads; i++) {
thread_ids[i] = i + 1;
pthread_create(&threads[i], NULL, &thread_func, &thread_ids[i]);
}
// Wait for threads to finish
for (i = 0; i < num_threads; i++) {
pthread_join(threads[i], NULL);
}
// Destroy the spinlock
pthread_spin_destroy(&lock);
return 0;
}
```
该程序使用 pthread 库中的自旋锁。在主函数中,它创建指定数量的线程,并将每个线程的 ID 传递给线程函数。线程函数尝试获取锁并保持 5 秒钟,然后释放锁。在 shell 脚本中,它使用 make 编译 C 代码并运行程序,最后清理生成的文件。
相关推荐
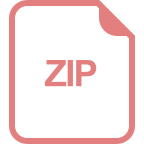
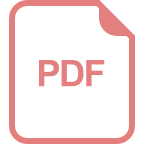
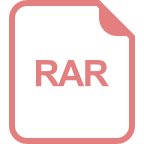












