window7 tail -f
时间: 2023-10-03 08:01:04 浏览: 99
"tail -f" 是一个在Window7操作系统中的命令行命令,用于实时监视和查看文件的变化。它的功能类似于Linux系统中的"tail -f"命令。
在Window7中,使用"tail -f"命令需要借助于其他的工具或软件来实现。以下是一种方法:
首先,在Window7操作系统中安装一个支持命令行工具的软件,例如Cygwin或Git Bash。这些软件提供了类似于Linux系统的命令行环境。
安装完成后,打开命令行窗口,并进入到需要监视的文件所在的目录。
然后,在命令行中输入"tail -f 文件名"命令,其中"文件名"是需要实时监视的文件的名称(包括路径)。
接下来,按下回车键,命令行窗口会开始实时显示文件的内容,并持续更新显示新添加的内容。
通过使用"tail -f"命令,可以方便地监视日志文件、系统日志等持续变化的文件。在命令行窗口中,可以通过按下Ctrl + C组合键来停止实时监视。
需要注意的是,以上方法只是一种实现"tail -f"功能的方式,具体的操作可能会因使用的软件或环境而有所不同。如果有其他工具或软件提供了更直接的"tail -f"功能,也可以选择使用。
相关问题
#! /system/bin/sh # 监听按键的设备文件和按键值 DEVICE_FILE="/dev/input/event0" KEY_VALUE="00a5" KEY_VALUE2="00a3" DISPLAY_ID=$(dumpsys window | awk '/displayId=/ {print $1}' | sed 's/displayId=//g') local id1=$(echo $DISPLAY_ID | awk '{print $1}') local id2=$(echo $DISPLAY_ID | awk '{print $2}') # 按键副屏打开 function key_state() { if [ "$id1" -gt "$id2" ]; then echo $id1 am start -n com.tencent.start.tv/com.tencent.start.ui.SplashActivity --display "$id1" else echo $id2 am start -n com.tencent.start.tv/com.tencent.start.ui.SplashActivity --display "$id2" fi } # 监听按键事件 function listen_key_events() { # 判断设备文件是否存在 if [ ! -e "$DEVICE_FILE" ]; then echo "Error: Device file '$DEVICE_FILE' does not exist." exit 1 fi # 监听按键事件 getevent -q "$DEVICE_FILE" | while read line; do local keycode=$(echo "$line" | awk '{print $2}') local value=$(echo "$line" | awk '{print $3}') local id_activity=$(dumpsys window | grep display | grep 'imeInputTarget' | awk '{print $4,$7}' | cut -d'}' -f1) local focus=$(dumpsys window | grep "mCurrentFocus" | tail -n 1 | awk '{print $NF}' | cut -d'}' -f1) local activity=$(echo "$id_activity" | grep "$focus"| awk '{print $NF}') if [ "$keycode" = "$KEY_VALUE" ]; then if [ "$value" = "00000001" ]; then key_state fi elif [ "$keycode" = "$KEY_VALUE2" ]; then if [ "$value" = "00000001" ]; then if [ "$activity" = "$(echo $id_activity | awk '{print $2}')" ] then echo "$(echo $id_activity | awk '{print $4}')" am start -n "$(echo $id_activity | awk '{print $4}')" --display "$(echo $id_activity | awk '{print $3}')" else echo "$(echo $id_activity | awk '{print $2}')" am start -n "$(echo $id_activity | awk '{print $2}')" --display "$(echo $id_activity | awk '{print $1}')" fi fi fi done } # 启动按键监听 listen_key_events 优化
这是一个监听按键事件的脚本,主要用于判断用户是否按下了指定的按键,如果是,则根据一定的逻辑打开相应的应用或操作。在优化方面,可以考虑以下几点:
1. 使用变量存储常量,方便修改和维护。例如,将设备文件和按键值的值存储在变量中,避免硬编码。
2. 在判断设备文件是否存在时,使用if加上-f参数判断文件是否存在,这样更简洁。
3. 在获取焦点窗口和当前活动窗口时,使用dumpsys的时候可以加上-grepto参数,只获取我们需要的信息,避免不必要的输出。
4. 在判断打开应用的逻辑时,可以使用case语句代替if-else语句,更加简洁易读。
5. 在启动按键监听之前,可以加上一些初始化操作,例如设置环境变量等。
综上所述,我们可以对脚本进行如下优化:
#! /system/bin/sh
# 常量定义
DEVICE_FILE="/dev/input/event0"
KEY_VALUE="00a5"
KEY_VALUE2="00a3"
# 初始化操作
DISPLAY_ID=$(dumpsys window | awk '/displayId=/ {print $1}' | sed 's/displayId=//g')
ID1=$(echo $DISPLAY_ID | awk '{print $1}')
ID2=$(echo $DISPLAY_ID | awk '{print $2}')
# 按键副屏打开
function key_state() {
if [ "$ID1" -gt "$ID2" ]; then
echo $ID1
am start -n com.tencent.start.tv/com.tencent.start.ui.SplashActivity --display "$ID1"
else
echo $ID2
am start -n com.tencent.start.tv/com.tencent.start.ui.SplashActivity --display "$ID2"
fi
}
# 监听按键事件
function listen_key_events() {
# 判断设备文件是否存在
if [ ! -f "$DEVICE_FILE" ]; then
echo "Error: Device file '$DEVICE_FILE' does not exist."
exit 1
fi
# 监听按键事件
getevent -q "$DEVICE_FILE" | while read line; do
local keycode=$(echo "$line" | awk '{print $2}')
local value=$(echo "$line" | awk '{print $3}')
# 获取焦点窗口和当前活动窗口
local id_activity=$(dumpsys window | grep -E 'mCurrentFocus|imeInputTarget' | grep 'id=' | awk '{print $1,$4}' | cut -d'}' -f1)
local focus=$(echo "$id_activity" | grep 'mCurrentFocus' | awk '{print $NF}' | cut -d'}' -f1)
local activity=$(echo "$id_activity" | grep 'imeInputTarget' | awk '{print $NF}')
case "$keycode" in
"$KEY_VALUE")
if [ "$value" = "00000001" ]; then
key_state
fi
;;
"$KEY_VALUE2")
if [ "$value" = "00000001" ]; then
if [ "$activity" = "$(echo $id_activity | awk '{print $2}')" ]; then
echo "$(echo $id_activity | awk '{print $4}')"
am start -n "$(echo $id_activity | awk '{print $4}')" --display "$(echo $id_activity | awk '{print $3}')"
else
echo "$(echo $id_activity | awk '{print $2}')"
am start -n "$(echo $id_activity | awk '{print $2}')" --display "$(echo $id_activity | awk '{print $1}')"
fi
fi
;;
esac
done
}
# 启动按键监听
listen_key_events
Write java code: Copy the files, small_weapons.txt, and large_weapons.txt from the assignment folder on Blackboard and save them to your folder. For testing purposes, you should use the small file. Use the large file when you think the application works correctly. To see what is in the files use a text editor. Nilesh is currently enjoying the action RPG game Torchlight 2 which is an awesome game and totally blows Auction House Simulator 3, oh sorry, that should be Diablo 3, out of the water. He has got a file containing info on some of the unique weapons in the game. The transaction file contains the following information: Weapon Name (string) Weapon Type (string) Damage (int) Weapon Speed (double) … To tell if one weapon is better than another you need to know the Damage Per Second (DPS) the weapon does, since weapons have a different attack speed. DPS is calculated by taking the damage value and dividing it by the attack speed.a) You will write a program that will allow Nilesh to load the file and display the weapon info and DPS of every weapon in the input file. When the user chooses to open a weapon file, they are required to type in the filename. It will then read the 4 values about a particular weapon and then display the 4 values to the console window on one line neatly padded along with the DPS of the weapon. This is repeated until the end of the file. b) Modify your code from a) so that the weapon information written to the console window is also written to a text file. The user should be able to specify the name of the file. Add a header to the beginning of the file which has column headers for each column. At the end of the text file display the total number of weapons in the file. c) Document your code appropriately and add your name and id number as comments at the top of your code. Please also submit this text file you have created. Enter the file you want to search end with .txt: large_weapons.txt 1 Blackfang Bludgeon Great Hammer 489 1.44 339.58333333333337 2 Bones 2 Boneshredder Great Axe 256 0.84 304.76190476190476 3 Comet's Tail Great Sword 872 1.2 726.6666666666667 4 Decapitator Great Sword 188 1.08 174.07407407407408 5 Demolisher Great Hammer 887 1.32 671.9696969696969
```
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
import java.util.Scanner;
public class WeaponInfo {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter the file you want to search end with .txt: ");
String fileName = scanner.nextLine();
File file = new File(fileName);
try {
Scanner fileScanner = new Scanner(file);
FileWriter writer = new FileWriter(new File("weapon_info_output.txt"));
writer.write("Weapon Name\tWeapon Type\tDamage\tWeapon Speed\tDPS\n");
int count = 0;
while (fileScanner.hasNextLine()) {
String line = fileScanner.nextLine();
String[] tokens = line.split(" ");
String weaponName = tokens[0];
String weaponType = tokens[1];
int damage = Integer.parseInt(tokens[2]);
double weaponSpeed = Double.parseDouble(tokens[3]);
double dps = damage / weaponSpeed;
System.out.printf("%-20s%-20s%-10d%-15.2f%.2f\n", weaponName, weaponType, damage, weaponSpeed, dps);
writer.write(weaponName + "\t" + weaponType + "\t" + damage + "\t" + weaponSpeed + "\t" + dps + "\n");
count++;
}
System.out.println("Total number of weapons: " + count);
writer.write("Total number of weapons: " + count);
writer.close();
fileScanner.close();
} catch (IOException e) {
System.out.println("Unable to read file: " + fileName);
} catch (NumberFormatException e) {
System.out.println("Invalid file format: " + fileName);
}
scanner.close();
}
}
```
阅读全文
相关推荐








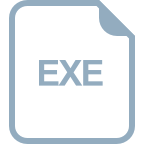



