C++怎么自动读取数据目录下新增的库文件,以支持mod加载
时间: 2024-12-11 13:31:27 浏览: 4
在C++中,自动读取数据目录(通常是程序安装路径下的某个资源文件夹)下新添加的库文件通常涉及到动态链接库(DLLs)或共享对象(SOs)。为了实现在运行时动态加载新的模块,你可以使用`dlopen`函数,这是POSIX标准的一部分,但在Windows上可以使用`LoadLibrary`或`GetProcAddress`。
以下是一个基本示例:
```cpp
#include <dlfcn.h> // Windows: #include <windows.h>
#include <string>
// 定义一个结构体,代表你想要从库文件加载的功能
struct LibraryFunction {
void (*function_name)(int param);
};
// 动态加载库函数
void* load_library(const std::string& library_path) {
void* handle = dlopen(library_path.c_str(), RTLD_LAZY); // Linux/Unix
// Windows: HMODULE handle = LoadLibraryA(library_path.c_str());
if (!handle) {
std::cerr << "Error loading library: " << dlerror() << std::endl; // 或者 GetLastError() for Windows
return nullptr;
}
// 搜索你需要的函数
LibraryFunction* function = (LibraryFunction*)dlsym(handle, "function_name"); // Linux/Unix
// Windows: function = (LibraryFunction*)GetProcAddress(handle, "function_name");
if (!function) {
dlclose(handle); // 或者 FreeLibrary(handle)
std::cerr << "Function not found in the library" << std::endl;
return nullptr;
}
return function->function_name;
}
int main() {
std::string lib_dir = "path_to_your_data_directory";
std::string new_library = "new_library.dll"; // 或者.so for Unix systems
// 遍历目录寻找新库
for (const auto& file : /* iterate over files in directory */) {
if (file == new_library) {
void* loaded_function = load_library(lib_dir + "/" + file);
if (loaded_function) {
(*loaded_function)(42); // 调用加载的函数
}
}
}
return 0;
}
```
阅读全文
相关推荐
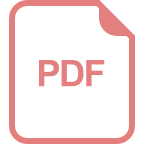
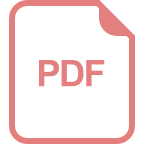
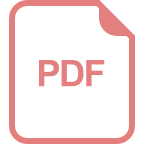
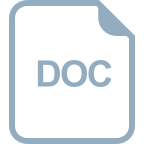
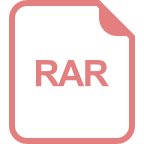
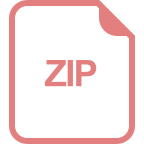
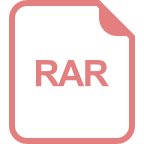
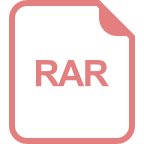
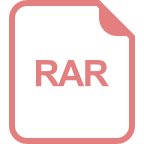
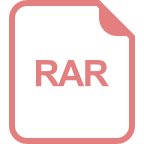
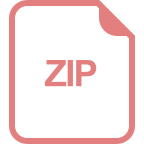
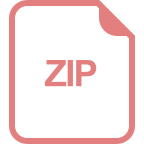
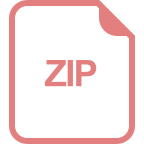
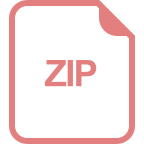
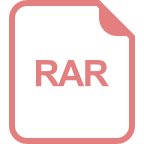