multimap insert
时间: 2024-10-31 21:07:15 浏览: 23
`multimap` 是 C++ 中的一个关联容器,它是 `std::map` 的多值版本,允许每个键关联多个值。`insert` 函数用于向 `multimap` 中添加新的元素对。它的基本语法如下:
```cpp
template <typename Key, typename T, typename Compare = std::less<Key>,
typename Alloc = std::allocator<std::pair<const Key, T>>>
void multimap<Key, T, Compare, Alloc>::insert(
iterator position,
const pair<const Key, T>& value);
```
`position` 参数是一个指向容器内部位置的迭代器,它决定了新插入的元素的位置。如果你想在容器的尾部插入,可以使用 `end()` 函数作为位置。
你可以通过如下方式插入新的元素:
```cpp
std::multimap<int, string> myMap;
// 插入一个新的整数和字符串对
myMap.insert(myMap.end(), std::make_pair(10, "hello")); // (10, "hello")
```
如果你尝试插入的键已经存在,并且你想添加另一个值,`insert` 会成功并增加该键对应的值的数量。
相关问题
multimap insert
multimap in C++ is a container that allows multiple values to be associated with a single key. To insert elements into a multimap, you can use the `insert` function.
Here's an example of how to use `insert` to add elements to a multimap:
```cpp
#include <iostream>
#include <map>
int main() {
std::multimap<int, std::string> myMultimap;
// Inserting elements using insert
myMultimap.insert(std::make_pair(1, "apple"));
myMultimap.insert(std::make_pair(2, "banana"));
myMultimap.insert(std::make_pair(2, "grape"));
myMultimap.insert(std::make_pair(3, "orange"));
// Print the multimap
for (const auto& pair : myMultimap) {
std::cout << pair.first << ": " << pair.second << std::endl;
}
return 0;
}
```
In this example, we create a multimap `myMultimap` with an integer key and a string value. We then use the `insert` function to add elements to the multimap. Note that the `insert` function takes a pair of key-value as its argument.
The output of the code above will be:
```
1: apple
2: banana
2: grape
3: orange
```
As you can see, the `insert` function allows us to add multiple values with the same key to the multimap.
multimap insert 已经存在的键 值
在C++标准库中,`std::multimap`是一个关联容器,它允许每个元素有一个或多值。当你尝试使用`insert`函数插入已存在的键对应的值时,`multimap`的行为取决于其内部的实现。如果键已经存在,`insert`操作会将新的值添加到该键对应的值集合中,而不是替换原有值。
例如:
```cpp
std::multimap<int, string> myMultimap;
myMultimap.insert({1, "apple"});
myMultimap.insert({1, "banana"}); // 尽管1已经被插入过,这里还会再添加"banana"
// 插入后,myMultimap[1]将是{ "apple", "banana" }
```
如果你不想增加现有的值,而是想替换它们,那么你应该先删除原有的键值对,然后再插入新值。可以使用`erase`和`insert`结合来实现:
```cpp
if (myMultimap.find(1) != myMultimap.end()) {
myMultimap.erase(myMultimap.find(1));
}
myMultimap.insert({1, "new_value"});
```
阅读全文
相关推荐
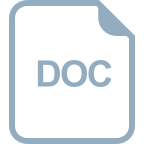
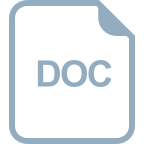
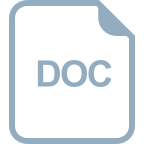
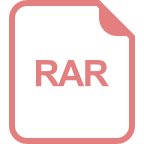












