HttpRequest设置Content-Type
时间: 2023-10-02 12:07:49 浏览: 164
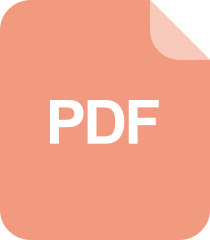
Content-type的说明即HTTP请求头的类型整理
你可以使用HttpRequest对象的setHeader()方法来设置Content-Type。示例代码如下:
```python
import requests
url = "http://example.com/api"
headers = {
"Content-Type": "application/json" # 设置Content-Type为application/json
}
data = {
"key": "value"
}
response = requests.post(url, headers=headers, json=data)
```
在上面的示例中,我们使用了requests库发送了一个POST请求,并设置了Content-Type为"application/json"。你可以根据需要修改Content-Type的值,例如"application/x-www-form-urlencoded"或"multipart/form-data"等。
阅读全文
相关推荐
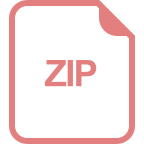

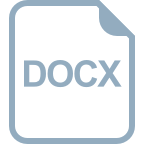
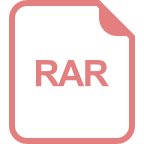
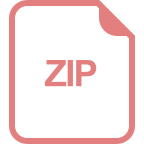
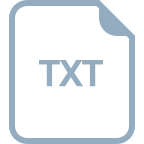
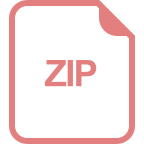
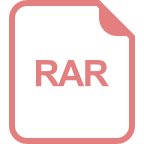







