用python实现一种智能优化算法
时间: 2024-10-22 18:09:41 浏览: 25
Python是一种非常适合实现各种智能优化算法的语言,因为它有着丰富的科学计算库如NumPy、SciPy和Pandas,以及强大的机器学习库如Scikit-Learn和TensorFlow。这里举一个简单的例子,我们可以使用遗传算法(Genetic Algorithm,GA)作为智能优化算法,它模拟了生物进化过程。
遗传算法通常包含以下几个步骤:
1. 初始化种群:创建一组随机生成的解,称为“个体”,每个个体代表一个可能的解决方案。
2. 适应度评估:给每个个体分配一个适应度值,这个值通常反映了该解对目标函数的好坏程度。
3. 选择操作:从当前种群中选择一些个体作为父母,基于他们的适应度来进行选择,概率较高的个体被选中的机会更大。
4. 繁殖:通过交叉(Crossover)和变异(Mutation)操作,生成下一代种群。交叉通常交换两个个体的部分基因,而变异则改变部分基因以增加多样性。
5. 重复:重复步骤2到4,直到达到预设的迭代次数,或者找到足够优秀的解。
以下是一个简化的遗传算法实现示例:
```python
import random
# 定义一个适应度函数和问题实例
def fitness_function(individual):
# 这里假设individual是优化的目标变量列表
return sum(individual)
def crossover(parent1, parent2):
# 随机交叉点
cut_point = random.randint(0, len(parent1) - 1)
child = parent1[:cut_point] + parent2[cut_point:]
return child
def mutation(child):
# 随机选择并修改一个元素
index = random.randint(0, len(child) - 1)
child[index] = random.randint(0, 100) # 假设我们优化的是整数数组
return child
# 主程序
def genetic_algorithm(population_size, generations, problem_size):
population = [random.sample(range(100), problem_size) for _ in range(population_size)]
for _ in range(generations):
parents = select(population, fitness_function)
children = []
for _ in range(int(population_size / 2)):
parent1, parent2 = random.choice(parents), random.choice(parents)
child = crossover(parent1, parent2)
child = mutation(child)
children.append(child)
population = combine(parents, children)
best_solution = max(population, key=fitness_function)
return best_solution, fitness_function(best_solution)
population_size, generations, problem_size = 100, 1000, 10
best_solution, fitness_value = genetic_algorithm(population_size, generations, problem_size)
print(f"Best solution found: {best_solution}, Fitness value: {fitness_value}")
阅读全文
相关推荐


















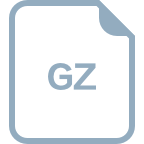