Unity2D代码实现点击在平面上的鱼群后于散开,之后再游向领头鱼
时间: 2024-04-16 20:24:09 浏览: 42
要实现在Unity中点击平面上的鱼群后鱼群散开并游向领头鱼,可以按照以下步骤进行:
1. 创建鱼的预制体:首先创建一个鱼的预制体,可以使用Unity的模型编辑器或导入自定义模型。
2. 添加组件:给鱼添加刚体组件和碰撞器组件,以便进行物理模拟。确保鱼的刚体组件启用了运动学选项,以便通过代码控制鱼的运动。
3. 编写鱼的移动脚本:创建一个脚本用于控制鱼的移动。在脚本中,可以定义鱼的速度和运动方向。
```csharp
using UnityEngine;
public class FishMovement : MonoBehaviour
{
public float speed = 5f; // 鱼的移动速度
private Rigidbody2D fishRigidbody;
private Vector3 targetPosition;
private bool isMoving = false;
void Start()
{
fishRigidbody = GetComponent<Rigidbody2D>();
}
void Update()
{
if (isMoving)
{
// 计算鱼的移动方向
Vector3 direction = (targetPosition - transform.position).normalized;
// 计算鱼的移动速度
Vector3 velocity = direction * speed * Time.deltaTime;
// 应用力到鱼的刚体上
fishRigidbody.velocity = velocity;
}
else
{
fishRigidbody.velocity = Vector3.zero;
}
}
public void MoveToPosition(Vector3 position)
{
targetPosition = position;
isMoving = true;
}
public void StopMoving()
{
isMoving = false;
}
}
```
4. 创建鱼群管理器脚本:创建一个脚本用于管理鱼群的行为。在脚本中,可以检测点击平面上的位置,并将该位置传递给鱼群中的每一只鱼。
```csharp
using UnityEngine;
public class FishSchoolManager : MonoBehaviour
{
public Transform leaderFish; // 领头鱼
public GameObject fishPrefab; // 鱼的预制体
public int fishCount = 10; // 鱼的数量
private GameObject[] fishArray;
void Start()
{
fishArray = new GameObject[fishCount];
for (int i = 0; i < fishCount; i++)
{
// 在场景中实例化鱼的预制体
GameObject fish = Instantiate(fishPrefab, transform.position, Quaternion.identity);
fishArray[i] = fish;
// 设置鱼的领头鱼和初始位置
FishMovement fishMovement = fish.GetComponent<FishMovement>();
fishMovement.leaderFish = leaderFish.gameObject;
fishMovement.MoveToPosition(transform.position);
}
}
void Update()
{
if (Input.GetMouseButtonDown(0))
{
// 检测点击平面上的位置
Vector3 mousePosition = Camera.main.ScreenToWorldPoint(Input.mousePosition);
mousePosition.z = 0f;
// 让鱼群散开并游向领头鱼
foreach (GameObject fish in fishArray)
{
FishMovement fishMovement = fish.GetComponent<FishMovement>();
fishMovement.MoveToPosition(mousePosition);
}
}
}
}
```
5. 创建一个平面对象:在场景中创建一个平面对象,作为鱼群的活动范围。
6. 创建一个领头鱼对象:在场景中创建一个领头鱼对象,并将其设置为鱼群管理器脚本(FishSchoolManager)中的leaderFish。
通过以上步骤,你可以实现点击平面上的鱼群后鱼群散开并游向领头鱼的效果。希望对你有所帮助!
相关推荐
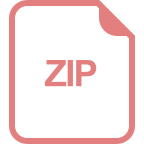
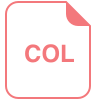













