有一个Excel表,里面包含海拔、纬度、经度,和15个光伏电站的名称,如何用python实现每个光伏之间的距离
时间: 2024-09-13 21:06:17 浏览: 64
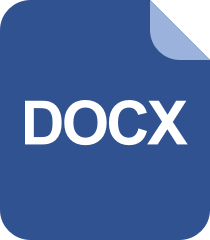
格尔木20MW光伏电站设计
要计算Excel表格中两个光伏电站之间的地理距离,你可以使用Python的pandas库处理数据,并结合geopy库来完成地理坐标的距离计算。以下是步骤:
1. **安装所需库**:
首先需要安装`pandas`、`openpyxl`(读取Excel文件)、`geopy`以及`numpy`库。你可以通过pip安装:
```bash
pip install pandas openpyxl geopy numpy
```
2. **加载数据**:
使用`pandas`读取Excel文件:
```python
import pandas as pd
# 假设文件名为'power_plants.xlsx'
df = pd.read_excel('power_plants.xlsx')
```
3. **提取必要的字段**:
确保你已经选择了正确的列,包括经纬度(例如`latitude`, `longitude`),如果它们不是默认的列名,替换为实际列名:
```python
df[['电站名称', '纬度', '经度']] # 如果有其他列名
```
4. **计算两点间的距离**:
使用`geopy`库中的`distance`模块,可以创建`Distance`对象,它会自动将经纬度转换为距离:
```python
from geopy.distance import great_circle
def calculate_distance(row):
station1 = (row['纬度'], row['经度'])
for index, other_row in df.iterrows():
if index != row.name and '电站名称' not in [row['电站名称'], other_row['电站名称']]: # 避免自相比较
station2 = (other_row['纬度'], other_row['经度'])
distance = great_circle(station1, station2).km
yield other_row['电站名称'], distance
distances = list(calculate_distance(df.iloc[0])) # 使用第一个电站开始计算
```
5. **将结果添加到DataFrame**:
将计算出的距离添加到原始DataFrame的新列,如`distance_to_others`:
```python
df['距离到其他电站'] = df.apply(lambda x: calculate_distance(x), axis=1)
```
6. **查看结果**:
最终的`df`应该包含了每个电站与其他所有电站的距离。
阅读全文
相关推荐
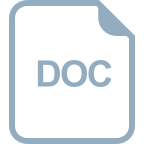
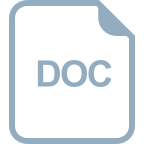

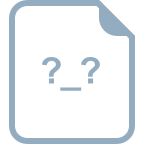
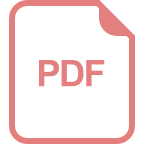
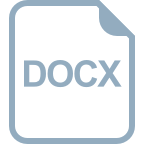
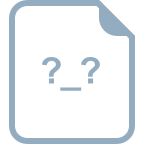
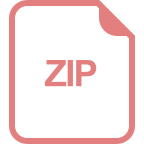
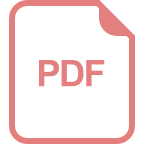
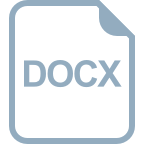
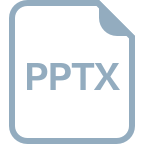
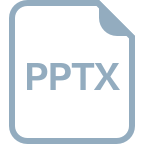
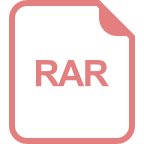
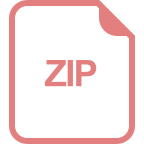
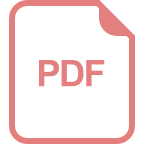
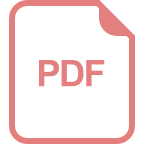
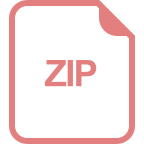